Compare two arrays and return the difference php
To implement a function similar to PHP’s array_diff without using built-in array functions, you can manually compare the values of two arrays. Here’s an example:
<?php
function customArrayDiff($array1, $array2) {
$difference = []; // Initialize an empty array to store differences.
foreach ($array1 as $value) {
// Check if the value is not in the second array.
$found = false;
foreach ($array2 as $checkValue) {
if ($value === $checkValue) {
$found = true;
break;
}
}
// If not found, add it to the difference array.
if (!$found) {
$difference[] = $value;
}
}
return $difference;
}
// Example usage
$array1 = [1, 2, 3, 4];
$array2 = [2, 4, 6];
$result = customArrayDiff($array1, $array2);
// Output the result
print_r($result);
?>
Output:
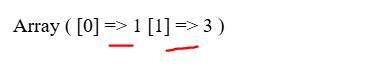
Array
(
[0] => 1
[1] => 3
)
Explanation:
- Initialize an Empty Array: Start with an empty array $difference to store the values that are in $array1 but not in $array2.
- Outer Loop: Loop through each element in $array1.
- Inner Loop: For each element in $array1, loop through $array2 to check if the current element exists in $array2.
- Flag for Match: Use a boolean flag ($found) to track if the value exists in $array2. If found, stop the inner loop.
- Add Unique Values: If the value is not found in $array2, add it to the $difference array.
- Return the Difference Array: After the loop, return the $difference array.
Keep Learning 🙂