Custom Implementation of array_diff_uassoc Without PHP Array Functions
To replicate the functionality of array_diff_uassoc in PHP without using PHP’s built-in array functions, you can create a custom function. This function compares two arrays by both key and value, using a user-defined callback function for comparison.
Here’s how you can do it:
Custom Implementation of array_diff_uassoc
<?php
function custom_array_diff_uassoc($array1, $array2, $keyCompareCallback) {
$result = [];
// Iterate through the first array
foreach ($array1 as $key1 => $value1) {
$keyExists = false;
// Iterate through the second array
foreach ($array2 as $key2 => $value2) {
// Use the custom callback to compare keys
if ($keyCompareCallback($key1, $key2) === 0 && $value1 === $value2) {
$keyExists = true;
break;
}
}
// If no matching key and value found, add to result
if (!$keyExists) {
$result[$key1] = $value1;
}
}
return $result;
}
// Example callback for case-sensitive key comparison
$keyCompareCallback = function($key1, $key2) {
return strcmp($key1, $key2); // Case-sensitive comparison
};
// Example usage
$array1 = ['a' => 1, 'b' => 2, 'c' => 3];
$array2 = ['b' => 2, 'd' => 4];
$difference = custom_array_diff_uassoc($array1, $array2, $keyCompareCallback);
print_r($difference);
?>
Output:
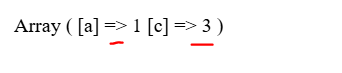
Array
(
[a] => 1
[c] => 3
)
Explanation:
- Input:
- $array1 and $array2: The arrays to compare.
- $keyCompareCallback: A user-defined function to compare keys.
- Iteration:
- The outer loop iterates over each key-value pair in $array1.
- The inner loop checks each key-value pair in $array2 for matches.
- Comparison:
- The keys are compared using the callback function.
- The values are compared with the === operator for strict comparison.
- Result Construction:
- If a key-value pair in $array1 does not have a match in $array2, it is added to the $result array.
- Output:
- The $result array contains the key-value pairs from $array1 that are not in $array2 based on the custom comparison.
Keep Learning 🙂