How to Build a PHP Function to Check Array Keys Without Using Built-In Functions
The array_key_exists function in PHP checks if a specific key exists in an array. Below is a custom implementation of this functionality without using PHP’s built-in array functions.
Code Example:
<?php
function custom_array_key_exists($key, $array) {
// Loop through the array keys
foreach ($array as $currentKey => $value) {
// Check if the current key matches the specified key
if ($currentKey === $key) {
return true; // Key exists
}
}
return false; // Key does not exist
}
// Example usage
$array = ['a' => 1, 'b' => 2, 'c' => 3];
$keyToCheck = 'b';
if (custom_array_key_exists($keyToCheck, $array)) {
echo "The key '$keyToCheck' exists in the array.";
} else {
echo "The key '$keyToCheck' does not exist in the array.";
}
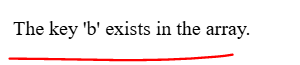
Explanation:
- Input Parameters:
- $key: The key to check for in the array.
- $array: The array to search in.
- Loop Through the Array:
- Use a foreach loop to iterate over the array.
- Compare each key ($currentKey) with the specified key ($key) using a strict comparison (===).
- Return Value:
- If a match is found, return true.
- If the loop completes without finding a match, return false.
Keep Learning 🙂