How to create the investment calculator in React JS
Steps:
a) Create “Components” folder inside src
b) Create Header.jsx component for title and header detail
c) Create UserInput.jsx component for input form
d) Create CalculateInvestment.jsx component for investment calculation function
e) Create ReceiveResult.jsx component for receive calculation output
Header Component: Inside the header component create title of project
return <header>
<h2>Investment Calculator</h2>
</header>
}
UserInput Component: is used for creating input form with different fields
return <section>
<div className="row">
<div className="column" >
<label>Monthly Investment</label>
<input type="text" value={userDetail.monthlyinvestment} onChange={(event) => onChange('monthlyinvestment', event.target.value)}/>
</div>
<div className="column" >
<label>Expected Return Rate annual %</label>
<input type="text" value={userDetail.expectedanualreturn} onChange={(event) => onChange('expectedanualreturn', event.target.value)}/>
</div>
</div>
<div className="row">
<div className="column" >
<label>Time Periods</label>
<input type="text" value={userDetail.timeduration} onChange={(event) => onChange('timeduration', event.target.value)}/>
</div>
</div>
</section>
}
CalculateInvestment Component: is used for getting the user input detail and for calculation
const annualData = [];
const totalInvestmenttime = timeduration * 12;
const totalInvestmentAmount = monthlyinvestment * totalInvestmenttime;
const decimal = expectedanualreturn / 100;
const rate = decimal / 12;
const compoundInterestFactor = Math.pow(1 + rate, totalInvestmenttime);
const numerator = compoundInterestFactor - 1;
const fraction = numerator / rate;
const totalMatureAmount = Math.round(monthlyinvestment * fraction * (1 + rate));
ReceiveResult Component: Inside ReceiveResult component receive the final result and render the detail
return <>
<div className="row">
<div className="resultcolumn" >
<p>Total Amount: {receivecalculateData.totalMatureAmount.toLocaleString()}</p>
<p>Investment Amount: {receivecalculateData.totalInvestmentAmount.toLocaleString()}</p>
<p>Est Return: {receivecalculateData.estReturn.toLocaleString()}</p>
</div>
</div>
</>
}
App Component:
Call all component inside app component and crate useState, handlefunction for hold input form values on change
import Header from './Components/Header';
import UserInput from './Components/UserInput';
import ReceiveResult from './Components/ReceiveResult';
const [userDetail, setUserDetail] = useState({
monthlyinvestment:20000,
expectedanualreturn:15,
timeduration:10
})
function handleChange(inputidentifier, usernewvalue){
setUserDetail(prev => {
return {
...prev,
[inputidentifier]: usernewvalue
}
})
}
Output:
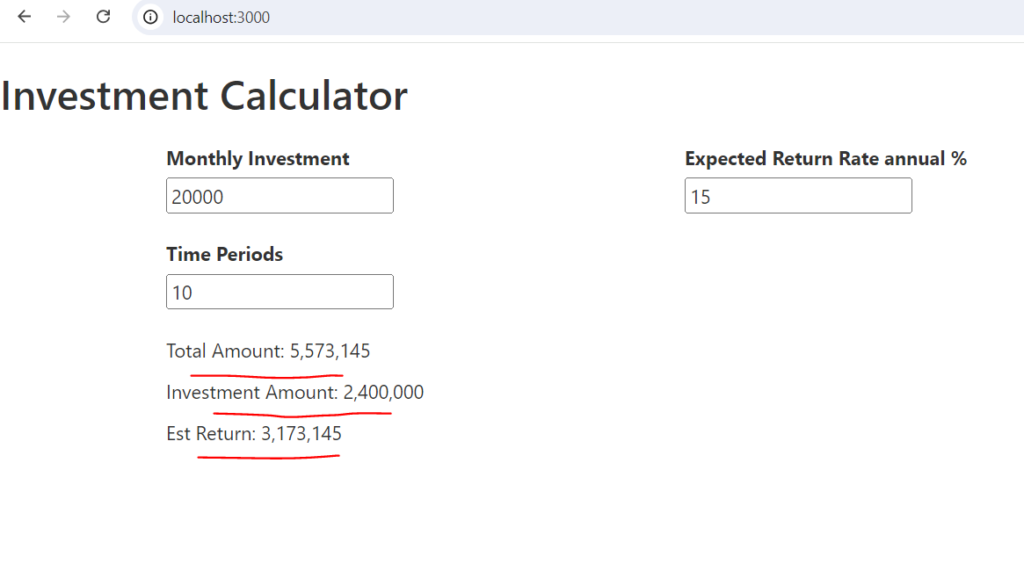
Complete Code:
App.jsx
import { useState } from 'react';
import Header from './Components/Header';
import UserInput from './Components/UserInput';
import ReceiveResult from './Components/ReceiveResult';
import './App.css';
const App = () =>{
const [userDetail, setUserDetail] = useState({
monthlyinvestment:20000,
expectedanualreturn:15,
timeduration:10
})
function handleChange(inputidentifier, usernewvalue){
setUserDetail(prev => {
return {
...prev,
[inputidentifier]: usernewvalue
}
})
}
return (
<>
<Header/>
<UserInput userDetail={userDetail} onChange={handleChange}/>
<ReceiveResult input={userDetail}/>
</>
)
}
export default App;
Header.jsx
export default function Header(){
return <header>
<h2>Investment Calculator</h2>
</header>
}
UserInput.jsx
export default function UserInput({ onChange, userDetail }){
return <section>
<div className="row">
<div className="column" >
<label>Monthly Investment</label>
<input type="text" value={userDetail.monthlyinvestment} onChange={(event) => onChange('monthlyinvestment', event.target.value)}/>
</div>
<div className="column" >
<label>Expected Return Rate annual %</label>
<input type="text" value={userDetail.expectedanualreturn} onChange={(event) => onChange('expectedanualreturn', event.target.value)}/>
</div>
</div>
<div className="row">
<div className="column" >
<label>Time Periods</label>
<input type="text" value={userDetail.timeduration} onChange={(event) => onChange('timeduration', event.target.value)}/>
</div>
</div>
</section>
}
ReceiveResult.jsx
import CalculateInvestment from './CalculateInvestment'
export default function ReceiveResult({ input }){
const receivecalculateData = CalculateInvestment(input);
return <>
<div className="row">
<div className="resultcolumn" >
<p>Total Amount: {receivecalculateData.totalMatureAmount.toLocaleString()}</p>
<p>Investment Amount: {receivecalculateData.totalInvestmentAmount.toLocaleString()}</p>
<p>Est Return: {receivecalculateData.estReturn.toLocaleString()}</p>
</div>
</div>
</>
}
CalculateInvestment.jsx
export default function CalculateInvestment({
monthlyinvestment,
expectedanualreturn,
timeduration
}){
const annualData = [];
const totalInvestmenttime = timeduration * 12;
const totalInvestmentAmount = monthlyinvestment * totalInvestmenttime;
const decimal = expectedanualreturn / 100;
const rate = decimal / 12;
const compoundInterestFactor = Math.pow(1 + rate, totalInvestmenttime);
const numerator = compoundInterestFactor - 1;
const fraction = numerator / rate;
const totalMatureAmount = Math.round(monthlyinvestment * fraction * (1 + rate));
return { totalMatureAmount :totalMatureAmount,
totalInvestmentAmount: totalInvestmentAmount,
estReturn:totalMatureAmount-totalInvestmentAmount
}
}
Keep Learning 🙂