How to get input text value using useState in React JS
Using following steps we can get the value of input text using useState in React js
Import useState from React.
Initialize state and hold the value of input field.
Bind the state value to the input attribute’s value.
When the input value changes update the state using the event handler.
Example-:
import React, { useState } from 'react';
function GetInputBoxValue() {
// Initialize state to hold the input value
const [inputValue, setInputValue] = useState('');
// Update the state using an event handler
const handleInputChange = (event) => {
setInputValue(event.target.value);
};
return (
<div>
{/* Bind the state value to the input's value attribute */}
<input
type="text"
value={inputValue}
onChange={handleInputChange}
placeholder="Enter text here"
/>
<p>You entered: {inputValue}</p>
</div>
);
}
export default GetInputBoxValue;
Code explanation :
Import useState:
First import the useState from React.
import React, { useState } from ‘react’;
Initialize State:
const [inputValue, setInputValue] = useState(”);
Initialize State with the default empty string value, setInputValue function is used for update the state and inputValue variable is used to assign the change value of text field
Bind State to Input:
<input type="text" value={inputValue} onChange={handleInputChange} />
The inputValue variable is used to set the value of text field so its controled by react
handleInputChange function call on change event to handle the input value change
Update State with Input Value:
handleInputChange function is used to update the value for text field whenever user enter value inside input field and for retriew the value use event.target.value.
Output:
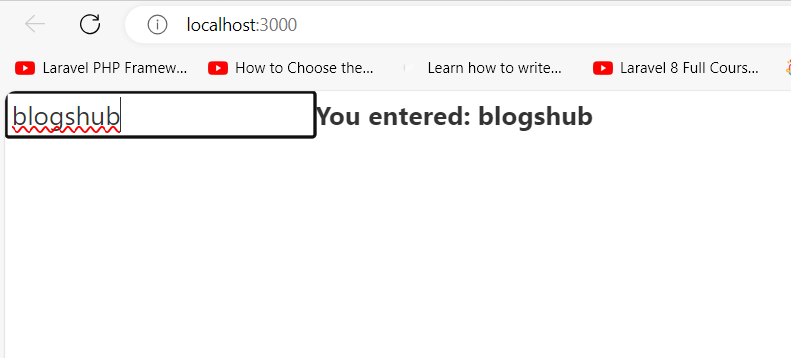
Keep Learning 🙂