How to upload image using CKEditor in PHP.
In this post we will learn how to upload using CKEditor with php from system.For image upload we will customize CKEditor library, we can download CKEditor library, and we can use cdn then we have to add filebrowserUploadUrl and need to create PHP file image upload functionality.
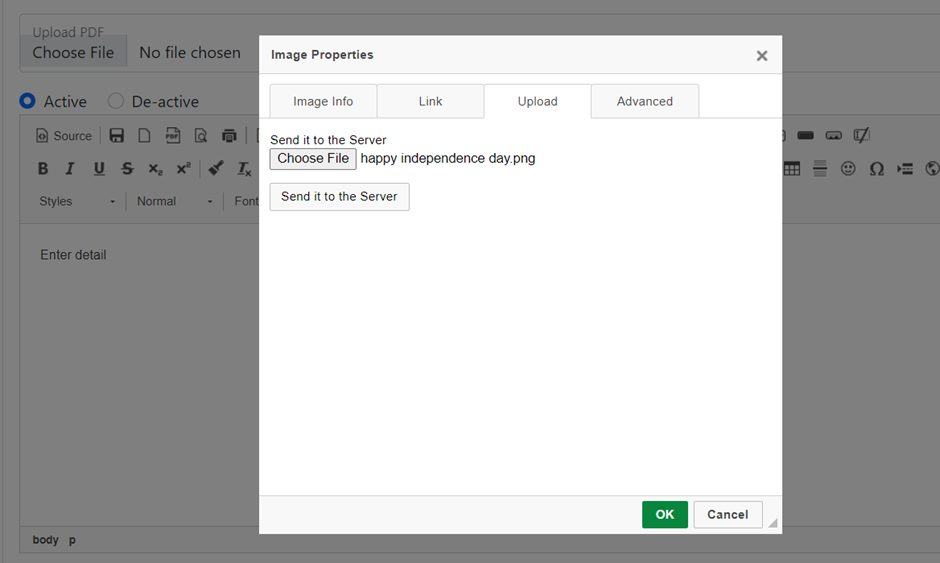
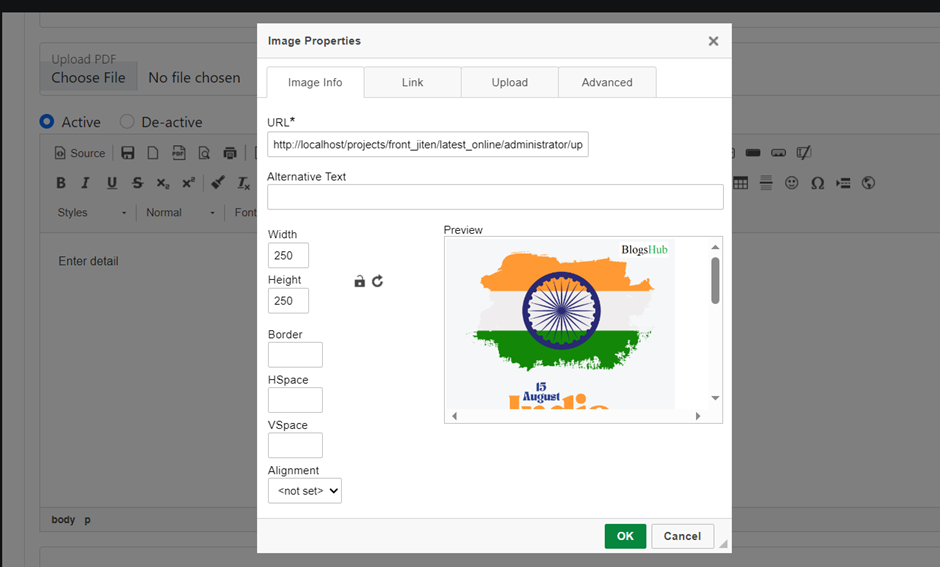
1) Download CKEditor library or use cdn
2) Replace HTML textarea with text editor.
3) Create upload directory for images.
4) Create PHP file for upload image functionality.
5) Add filebrowserUploadUrl in js for customize CKEditor feature.
example:
<textarea id="editor" name="editor">
<script src="https://ckeditor.com/latest/ckeditor.js"></script>
<script>
CKEDITOR.replace( 'editor', {
height: 300,
filebrowserUploadUrl: "upload.php",
filebrowserUploadMethod: 'form'
});
</script>
Complete Code:
index.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>How to upload image using CKEditor in PHP</title>
</head>
<body>
<h1>How to upload image using CKEditor in PHP.</h1>
<script src="https://ckeditor.com/latest/ckeditor.js"></script>
<form>
<label> Add Content </label> <br>
<textarea id="editor" name="editor">
<script>
CKEDITOR.replace( 'editor', {
height: 300,
filebrowserUploadUrl: "upload.php",
filebrowserUploadMethod: 'form'
});
</script>
</form>
</body>
</html>
upload.php
<?php
if(isset($_FILES['upload']['name']))
{
$file = $_FILES['upload']['tmp_name'];
$file_name = $_FILES['upload']['name'];
$file_name_array = explode(".", $file_name);
$extension = end($file_name_array);
$new_image_name =rand() . '.' . $extension;
if($extension!= "jpg" && $extension != "png" && $extension != "jpeg" && $extension != "PNG" && $extension != "JPG" && $extension != "JPEG") {
echo "<script type='text/javascript'>alert('Sorry, only JPG, JPEG, & PNG files are allowed. Close image properties window and try again');</script>";
}
elseif($_FILES["upload"]["size"] > 1000000) {
echo "<script type='text/javascript'>alert('Image is too large: Upload image under 1 MB . Close image properties window and try again');</script>";
}
else
{
move_uploaded_file($file, 'upload/' . $new_image_name);
$function_number = $_GET['CKEditorFuncNum'];
$HTTP_HOST = $_SERVER['HTTP_HOST'];
$url = "https://$HTTP_HOST/administrator/upload/".$new_image_name; //Set your path
// $url = "$HTTP_HOST/administrator/upload/".$new_image_name;
$message = '';
echo "<script type='text/javascript'>window.parent.CKEDITOR.tools.callFunction($function_number, '$url', '$message');</script>";
}
}
?>
Keep Learning 🙂