How to use useReducer hook in react js
useReducer: is used to manage sate in our react application, or we can say that its a state management tool. State Management is used to manage all application states in very simple way, whenever you have lot of states and method use useReducer to handle.
useReducer accespt two arguments one is reducer function which is used to mange all states and second is default state
Example:
import React, { useReducer } from 'react';
const ACTION = {
INCREASE : "increase",
DECREASE: "decrease"
}
const initialstate = {count: 0}
const reducer = (state, action) => {
console.log(action)
////return {count: state.count + 1}
switch(action.type){
case ACTION.INCREASE:
return {count: state.count + 1}
case ACTION.DECREASE:
return {count: state.count - 1}
default :
return state;
}
}
const App = () =>{
const [state, dispatch] = useReducer(reducer, initialstate)
const increaseCount = () => {
dispatch({type: ACTION.INCREASE})
//dispatch({type: "increase})
//setCount((prev) => prev +1);
};
const decreasCount = () => {
//dispatch({type: "decrease})
dispatch({type: ACTION.DECREASE})
//setCount((prev) => prev -1);
};
return (
<>
<div>
<p>Count : {state.count}</p>
<button onClick={increaseCount}>Increase</button>
<button onClick={decreasCount}>Decrease</button>
</div>
</>
)
}
export default App;
Output:
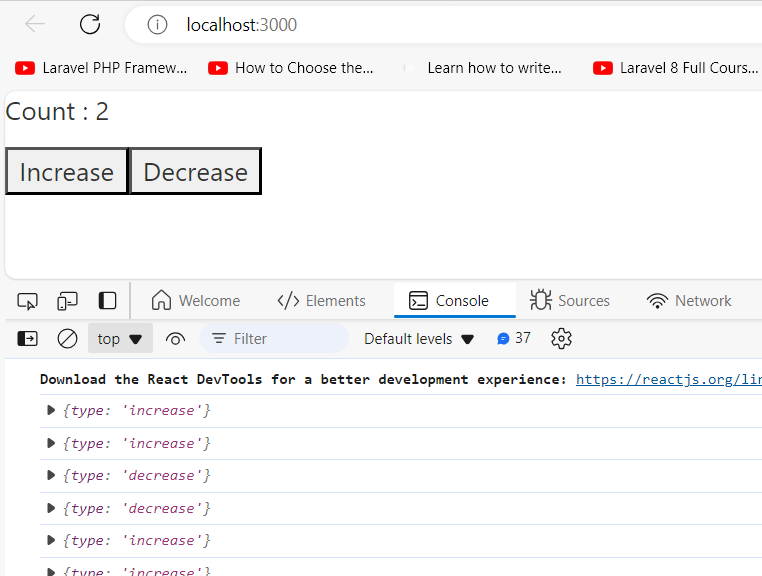
Keep Learning 🙂