Types Of Loops in JavaScript
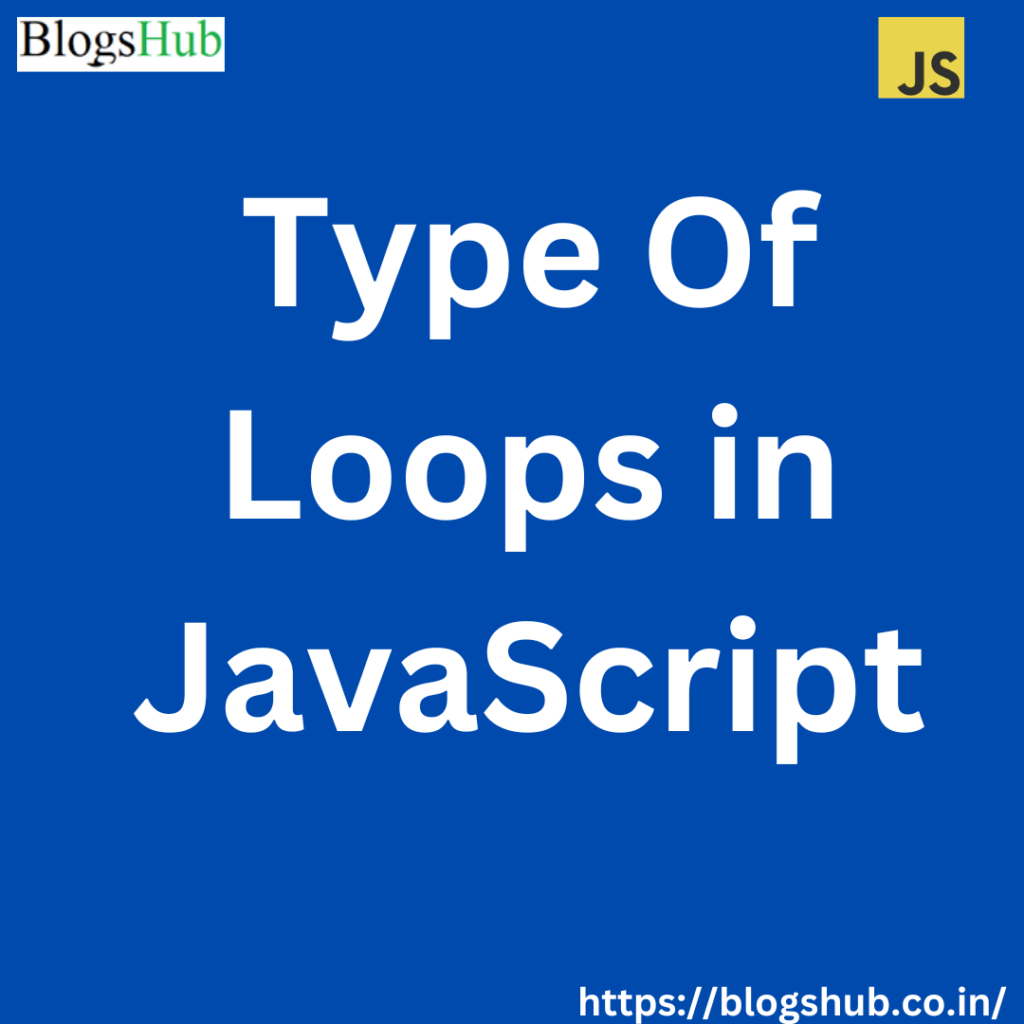
- For Loop
A loop that runs a block of code a specific number of times.
for (let i = 0; i < 15; i++) {
console.log(i);
}
Description: The classic loop, ideal when the number of iterations is known in advance.
2. While Loop
A loop that continues to execute as long as the condition is true.
let i = 0;
while (i < 15) {
console.log(i);
i++;
}
Description: A condition-controlled loop that runs until the condition becomes false.
3. do…while Loop
A loop that executes the block of code at least once, and then checks the condition.
let i = 0;
do {
console.log(i);
i++;
} while (i < 15);
Description: Always executes the code at least once, regardless of the initial condition.
4. For..of Loop
A loop that iterates over iterable objects like arrays.
let arr = [10, 20, 30, 15, 25 ,35];
for (let value of arr) {
console.log(value);
}
Description: Perfect for looping through arrays or other iterable objects.
5. for… in Loop
A loop that iterates over the properties of an object.
let obj = {a: 1, b: 2, c: 3, d: 10, e: 15};
for (let key in obj) {
console.log(key, obj[key]);
}
Description: Best suited for looping through object properties in key-value pairs.
keep Learning 🙂