What is ORM in Python
What is ORM : without query using inbuilt functions we can connect to db for fetching, inserting records
Sqlalchemy is ORM in Python
How to use ORM in Python
- Install Python: use below link for python installation
How to install Python in Window – BlogsHub
2. Install fastapi or any python framework: Using below link we can install fastapi
How to install fastapi in python – BlogsHub pip install fastapi
3. Install Sqlalchemy : Run below command
Reference : https://fastapi.tiangolo.com/tutorial/sql-databases/?h=sql
pip install sqlalchemy
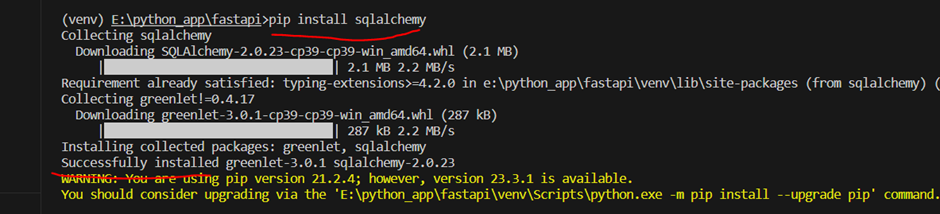
Import sqlalchemy in python file
from sqlalchemy.orm import Session
Create instance
app = FastAPI()
Create database file and import sqlchem engin and sessionmaker
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Create Database Connection
SQLALCHEMY_DATABASE_URL = 'postgresql://postgres:123456789@localhost/fastapi_ORM_DB'
engine = create_engine(SQLALCHEMY_DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Call Dependency
# Dependency
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
Create URL for access ORM
@app.get("/sqlalchemy")
async def test_post(db: Session = Depends(get_db)):
return {"MESSAGE": "test"}
Complete Code:
main.py
from typing import Optional
from fastapi import FastAPI, Response, status, HTTPException, Depends
from fastapi.params import Body
from pydantic import BaseModel
from random import randrange
import psycopg2
from psycopg2.extras import RealDictCursor
import time
from sqlalchemy.orm import Session
from .import models
from .database import engine, SessionLocal, get_db
models.Base.metadata.create_all(bind=engine)
app = FastAPI()
@app.get("/test")
async def get_method():
return {"MSG": "Get Method"}
@app.get("/sqlalchemy")
async def test_post(db: Session = Depends(get_db)):
return {"MESSAGE": "test"}
database.py
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# SQLALCHEMY_DATABASE_URL = 'postgresql://<username>:<password>@<ip-address/hostname>/<database_name>'
SQLALCHEMY_DATABASE_URL = 'postgresql://postgres:123456789@localhost/fastapi_ORM_DB'
engine = create_engine(SQLALCHEMY_DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
# Dependency
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
models.php
from sqlalchemy import Column, Integer, String, Boolean
from sqlalchemy.sql.expression import null
from .database import Base
class Post(Base):
__tablename__ = "post"
id = Column(Integer, primary_key=True, nullable=False)
title = Column(String, nullable=False)
content = Column(String, nullable=False)
published = Column(Boolean, default=True)
Run pyhon application:
uvicorn main:app --reload
Check your database for new post table
Keep Learning 🙂