What is props in react js
In react we can use “props” for passing data from parent to child component, props is the short of ‘properties’. Props are read only , read only means inside the child components props values can’t be change which value we are receive same we have to use, the props data control by the parent component only , child component use that data for render on frontend.
Working process of props:
Inside the component we can pass the props like attribute on html element and it (props) received by child component as an argument, its (props) allow component to be reusable with different data.
import React from 'react';
// Child Component
function Propsexample(props) {
return <h4>Here is {props.title}!</h4>;
}
// Parent Component
function App() {
return (
<div>
{/* Passing props to the child component */}
<Propsexample title="Props Content one" />
<Propsexample title="Props Content Two" />
<Propsexample title="Props Content Three" />
</div>
);
}
export default App;
Code Explanation:
Child Component which is Propsexample:
The Propsexample component receives props as an argument.
It uses props.title to display the title passed from the parent component.
For example, if props.title is “Props Content one”, the rendered output will be “Here is Props Content one!”.
Parent Component (App):
The App component renders three Propsexample components.
It passes different title props like: “Props Content one”,”Props Content two”,”Props Content three” to each Propsexample component.
Each Propsexample component renders a personalized message on the title prop it receives.
Key Points:
Reusability: We can reuse props by passing different arguments.
Read Only: Props are readonly, inside the child component we can only read the data we can’t modify it.
Output:
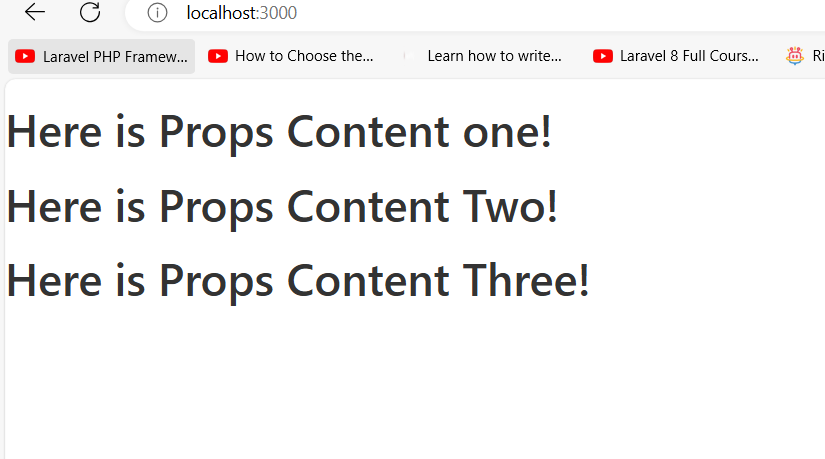
Keep Learning 🙂