Javascript Shorthand Methods
a. Optional Chaining
Long Method
const student = {
name: "BlogsHub",
address: {
street: "Hennur Road",
city: "Bangalore",
state: "Karnataka"
}
};
if (student && student.address && student.address.state){
//write your logic here
console.log(student.address.state)
}
Short Method
console.log(student?.address?.state)
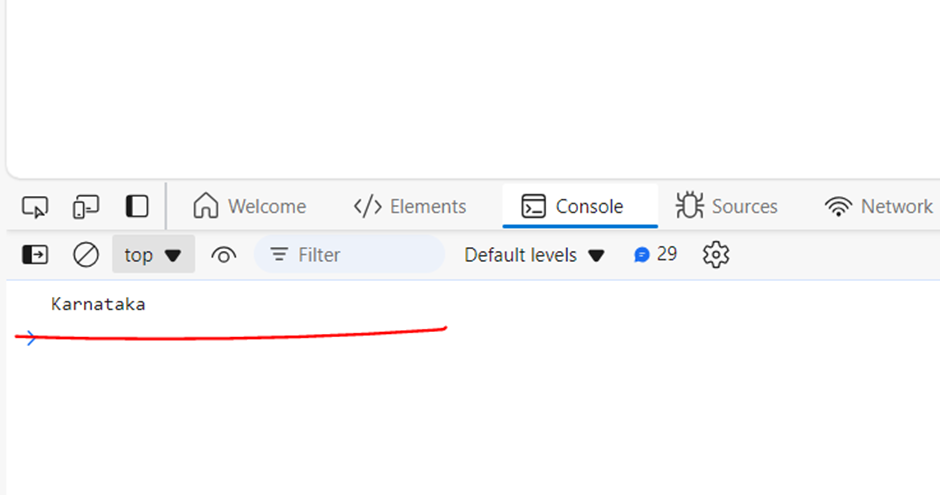
b. Spread Operator
Long Method
let firstsemMarks = [70, 60, 85]
let secondsemMarks = [75, 65, 94]
let totalMarks = firstsemMarks.concat(secondsemMarks)
console.log(totalMarks)
Short Method
let firstsemMarks = [70, 60, 85]
let secondsemMarks = [75, 65, 94]
let totalMarks =[...firstsemMarks, ...secondsemMarks]
console.log(totalMarks)
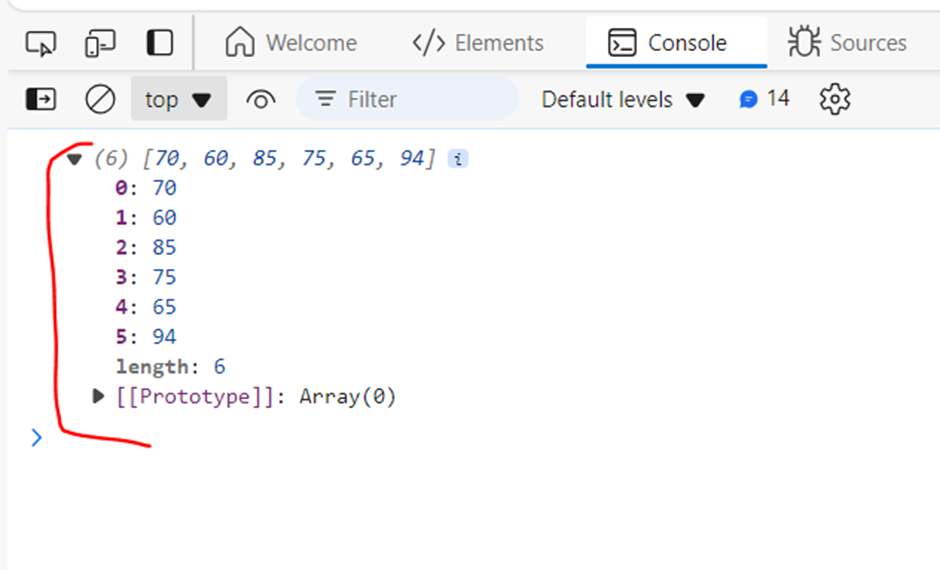
c. Array Destructuring
Long Method
let marks = [65, 75, 80, 85, 66]
let firstSubj = marks[0]
let secondSubj = marks[1]
let thirdSubj = marks[2]
console.log("firstSubj = ", firstSubj, "secondSubj = ", secondSubj, "thirdSubj = ", thirdSubj)
Short Method
let [firstSubj, secondSubj, thirdSubj] = marks;
console.log("firstSubj = ", firstSubj, "secondSubj = ", secondSubj, "thirdSubj = ", thirdSubj)

d. Array Mapping
Long Method
let marks = [65, 75, 80, 85, 66]
let graceMarks = marks.map(function (n) {
return n + 5;
});
console.log("graceMarks = ", graceMarks)
Short Method
let graceMarks = marks.map(n => n + 5);
console.log("graceMarks = ", graceMarks)
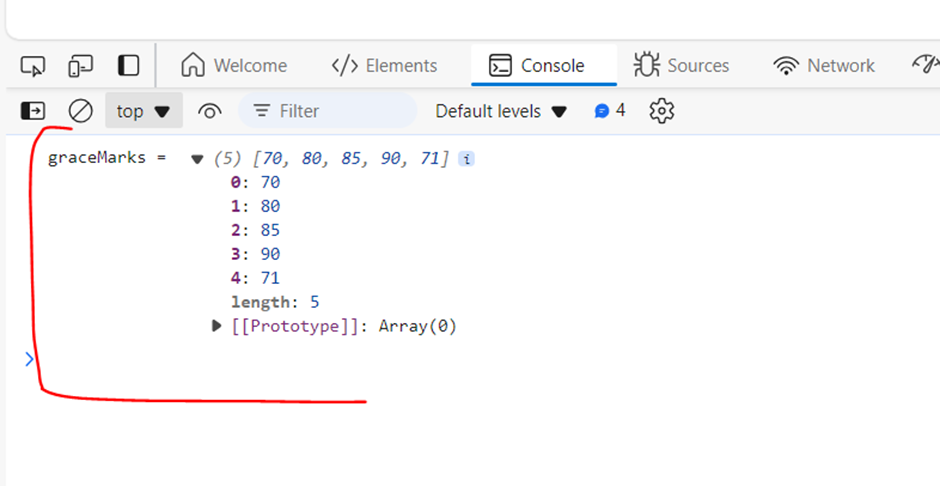
e. Filter array
Long Method
let marks = [65, 75, 80, 85, 66]
let greater = marks.filter(function(n){
return n > 75;
})
console.log(greater)
Short Method
let greater = marks.filter(n => n > 75);
console.log(greater)
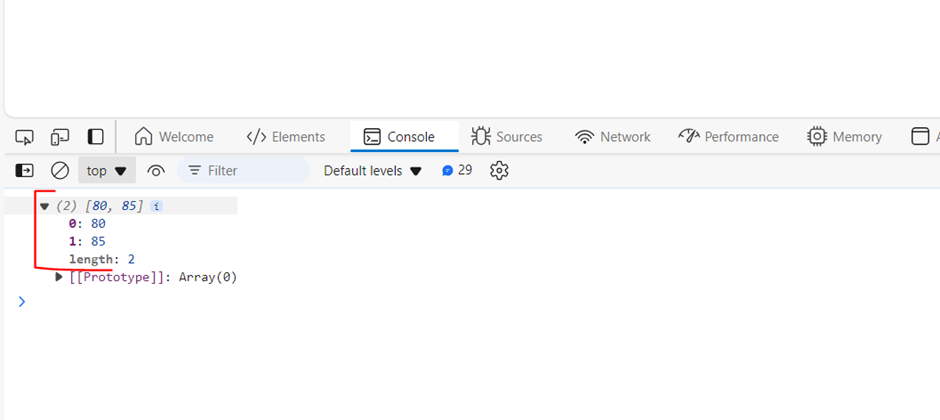
f. Swap Marks
Long Method
a = 5
b = 10
temp = a;
a =b ;
b = temp;
console.log("a", a , "b", b)
Short Method
[a, b] = [b, a];
console.log("a", a , "b", b)
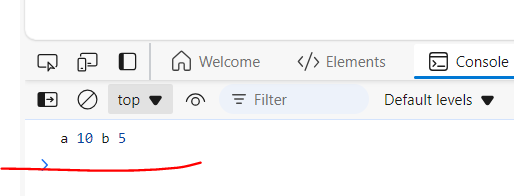
Keep Learning 🙂