Schema Validation with Pydantic in Python fastapi
Hey guys, in this post we will learn how to create the fields validation with API using Pydantic in Python fastapi.
- Import pydantic
- Create Class
- Create function with method (Post|PUT etc)
- Start webserver
- Run API in Postman
Import pydantic:
from pydantic import BaseModel
Create Class:
class Post(BaseModel):
title: str
description: str
Create Function:
@app.post("/createpost")
def createPost(new_post : Post):
print(new_post)
return {"Data": new_post}
Start Web Server:
uvicorn main:app --reload
Run API in Postman:
http://127.0.0.1:8000/createpost
Example with complete code:
from fastapi import FastAPI
from fastapi.params import Body
from pydantic import BaseModel
app = FastAPI()
class Post(BaseModel):
title: str
description: str
@app.post("/createpost")
def createPost(new_post : Post):
print(new_post)
return {"Data": new_post}
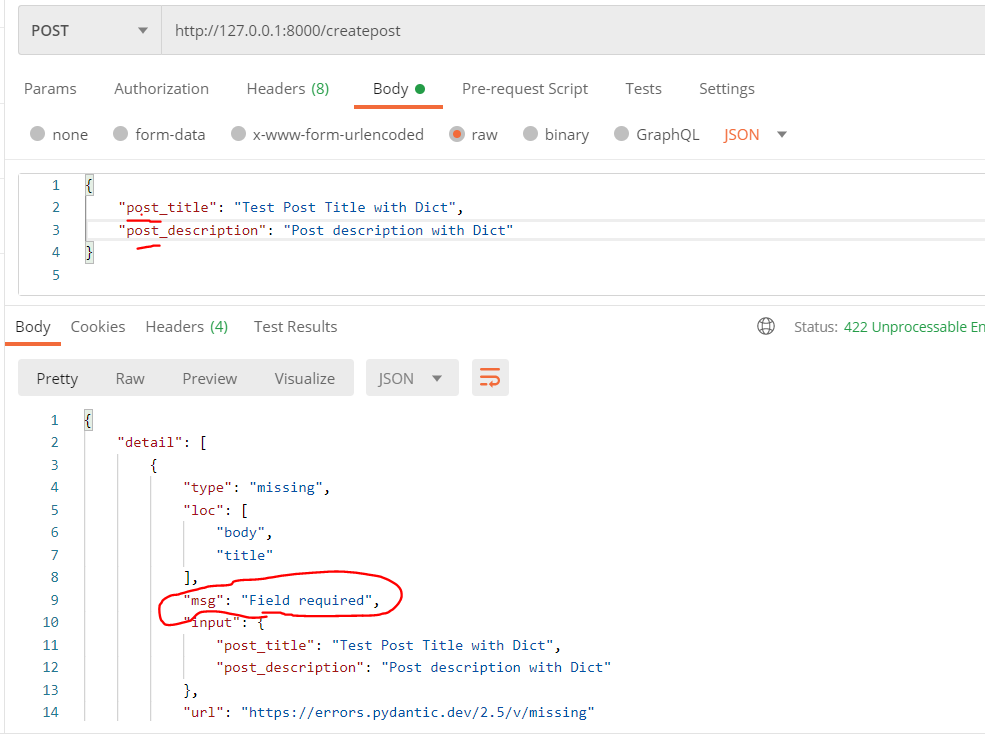
you can see in above example that in class Post title and description is using as a field but in postman body fields are (post_title, post_description) so on submit the api it will trough the “field require” error.
🙂 Keep Learning