File Handeling – open file in Perl
In this post, we will learn how to open the file in Perl. Using the open() function we will open the file.
We will also learn how to open the file for writing, reading and error handling using file.
Open function in Perl:
We use open() function with three arguments for open a file.
e.g., open(filehandle,mode,filename);
Filehandle: filehandle associates with the file.
Mode: we can open a file for writing, reading, and appending.
Filename: Third argument is filename using it we will set the path of file for open it.
With open() function we need to pass some operand which specify the mode of file to open.
e.g.
read <: In read mode we can only open the file we can’t change the content of file.
write >: In write mode we can write the content in file if file exist or if file does not exist a new file will create and then write the content and the content of file will wipe out.
append >> In append mode we can open the file and append the content, content will not wipe like write mode.
like: Syntex: open (FH, ‘<‘, ‘filepath’);
Example: open (FH, ‘<‘, ‘d:\myfile.txt’);
We can use the die () function to handle the failure of file function.
open (FH, ‘<‘, ‘d:\myfile.txt’) or die $!;
$! is used to convey the error message, it is a special variable which is used to tell why the open function fail.
File Closing:
After using file function like read, write and append we should close the file function using close() function, even Perl close the file automatically but for good practice we
should use close function always.
#!/usr/bin/perl
use warnings;
use strict;
my $filename = 'd:\myfile.txt';
open(FH, '<', $filename) or die $!;
print("File name $filename !\n");
close(FH);
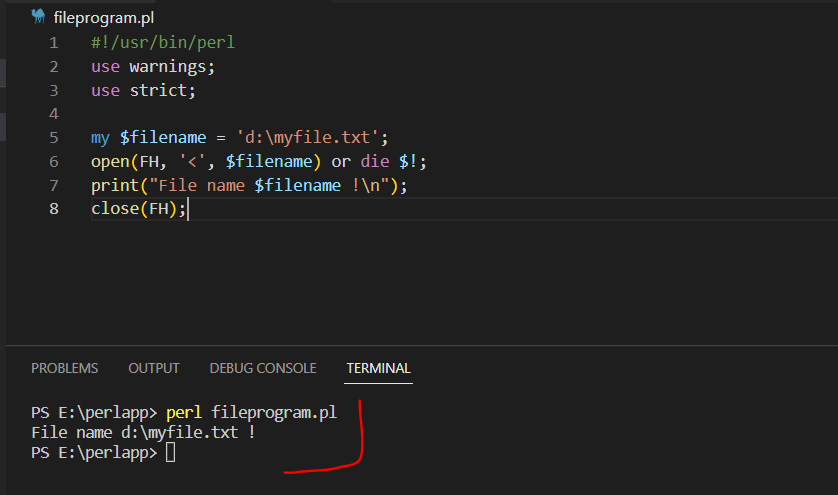
I needed to thank you for this wonderful read!! I absolutely loved every bit of it. I have you saved as a favorite to check out new things you post.