Full Stack Angular and Laravel CRUD Application Step by Step
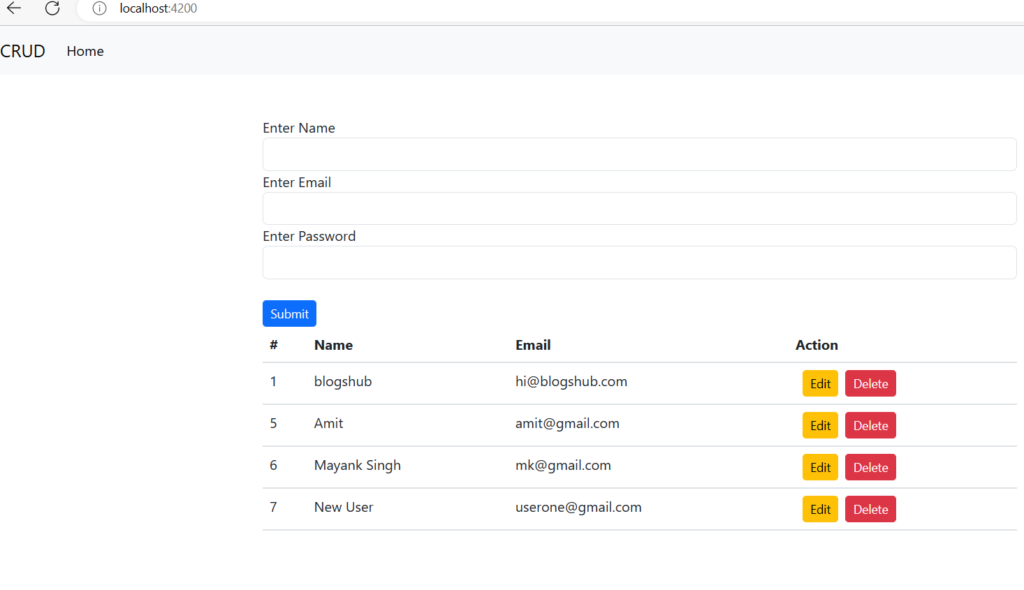
Hey Guys, in this post we will learn how to create CRUD (CREATE, READ, UPDATE and DELETE) application using Angular and Laravel API
for this application we will use laravel for backend and Angular for frontend
For installing Laravel and Creating CRUD API in Laravel you can follow below mention links
https://blogshub.co.in/how-to-install-laravel/
https://blogshub.co.in/create-laravel-crud-rest-api/
For creating restfull API you can follow above mention link , we will call these api in frontend .
So let install Angular first :
Install Angular : First we have to install angular using below mention npm command we can install angular , open command prompt and run below command then press enter.
npm install -g @angular/cli
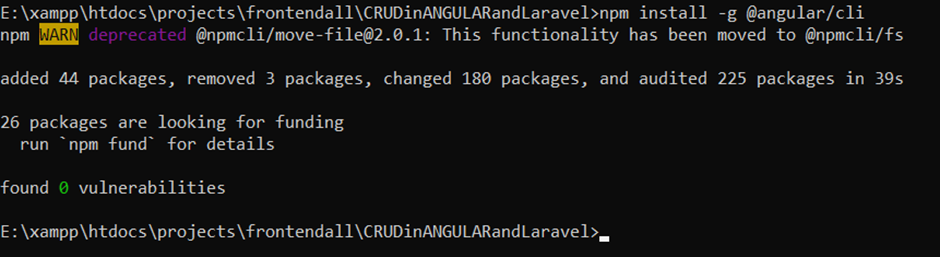
Create Project : Now we have to create new application or workspace, for that we have to run ng command as mention below.
ng new frontcrudapp
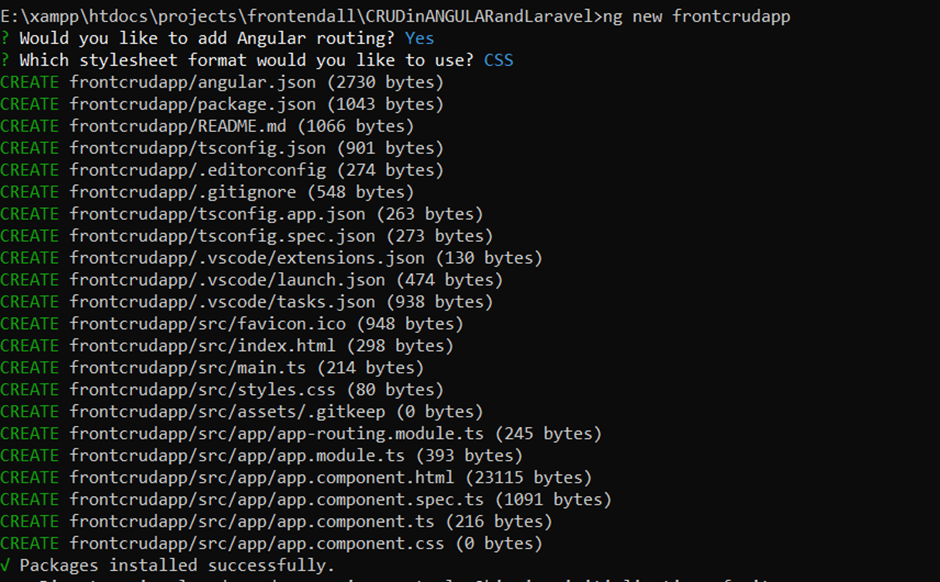
Now get in your application
cd frontcrudapp
Create Component: For CRUD steps and pages we have to create components , lets create component using below ng generate command
ng g c components/employee
Above command will create a one folder “components” and inside components folder it will create component files ,it will create five files .ts,.html and CSS files

Routes: routes are use for navigate the pages. For creating routes go inside app.module.ts and import routemodule, and then create route array and define routes path.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmployeeComponent } from './components/employee/employee.component';
import { NavbarComponent } from './components/navbar/navbar.component';
import { HttpClientModule } from '@angular/common/http';
import { FormsModule } from '@angular/forms';
import { EmployeeEditComponent } from './components/employee-edit/employee-edit.component';
const appRoutes : Routes = [
{path: '', component:EmployeeComponent},
{path: 'edit/:id', component:EmployeeEditComponent}
];
@NgModule({
declarations: [
AppComponent,
EmployeeComponent,
NavbarComponent,
EmployeeEditComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FormsModule,
RouterModule.forRoot(appRoutes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Services : For communicating components and API we have to create services inside services we will create some functions and using functions we will call API.
For creating services we will run the ng command in command prompt.
ng g s service/data

Open data service ts file for creating and accessing api
src\app\service\data.service.ts
import HttpClient
import { HttpClient } from '@angular/common/http';
create httpclient object inside construct function and create getData function for accessing API for fetching records.
constructor(private httpclient: HttpClient) { }
getData(){
return this.httpclient.get('http://localhost:8000/api/users/');
}
Create getData Function : For fetching the data to database we will create a function in service file.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private httpclient: HttpClient) { }
getData(){
return this.httpclient.get('http://localhost:8000/api/users/');
}
}
EmployeeComponent: open Employeecomponent ts file for defining array and function
(src\app\components\employee\employee.component.ts)
import { Component, OnInit } from '@angular/core';
import { DataService } from 'src/app/service/data.service';
@Component({
selector: 'app-employee',
templateUrl: './employee.component.html',
styleUrls: ['./employee.component.css']
})
export class EmployeeComponent implements OnInit {
// export class EmployeeComponent {
employees:any;
constructor(private dataService:DataService) { }
ngOnInit(){
this.getEmployeesdata();
}
getEmployeesdata() {
this.dataService.getData().subscribe(res =>{
this.employees = res;
});
}
}
Add bootstrap CSS in index.html
src\index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Frontcrudapp</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<app-root></app-root>
</body>
</html>
Navbar Component : Create a navbar component for creating a navbar in our application.
ng g c components/navbar

<nav class="navbar navbar-expand-lg bg-body-tertiary">
<div class="container-fluid">
<a class="navbar-brand" href="#">CRUD</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#">Home</a>
</li>
</ul>
</div>
</div>
</nav>
Fetch Data : Fetch data in employeecomponent html file
src\app\components\employee\employee.component.html
<table class="table">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let emp of employees">
<td>{{ emp.id }}</td>
<td>{{ emp.name }}</td>
<td>{{ emp.email }}</td>
</tr>
</tbody>
</table>
Insert Data :
For submit the data using angular we have to create a html form
So inside employee.component.html we will create a form
<form (ngSubmit)="insertData()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<div class="form-group">
<label for="name">Enter Password</label>
<input type="password" name="password" class="form-control" [(ngModel)]="employee.password">
</div>
<button class="btn btn-primary btn-sm mt-4">Submit</button>
</form>
Now we have to import the FormModule inside app.module.ts
src\app\app.module.ts
import { FormsModule } from '@angular/forms';
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FormsModule,
RouterModule.forRoot(appRoutes)
],
Then we have to call insertData function inside employee.component.ts, which we are using with HTML form.
src\app\components\employee\employee.component.ts
insertData(){
this.dataService.insertData(this.employee).subscribe(res =>{
this.getEmployeesdata();
});
Now create a model using below ng command in this model (employee.ts) we will define all input variable which we are getting through form like : name, email, password, its helpful for two way data binding, using [(ngModel)] we can pass these model data type in html form.
ng g class Employee

export class Employee {
name:any;
email:any;
password:any;
}
We have to call Employee model inside employee.component.ts for creating object
import { Employee } from 'src/app/employee';
employee = new Employee();
Now create insertData function in data.service.ts file for communicating with backend API
src\app\service\data.service.ts
insertData(data){
return this.httpclient.post('http://localhost:8000/api/users/',data);
}
Update Records :
For update records we have create a new component ,we can create a component in angular using ng command as below
ng g c components/employee-edit

Create edit button inside employee.component.html
<td> <button class="btn btn-warning btn-sm mx-2" routerLink="edit/{{ emp.id }}">Edit</button></td>
Now we have to create a edit route inside app.module.ts file
src\app\app.module.ts
const appRoutes : Routes = [
{path: '', component:EmployeeComponent},
{path: 'edit/:id', component:EmployeeEditComponent}
];
Create a functions in service file for fetching particular record by id and update records
src\app\service\data.service.ts
getEmployeeByID(id:any){
return this.httpclient.get('http://localhost:8000/api/users/'+id);
}
updateData(id:any,data:any){
return this.httpclient.put('http://localhost:8000/api/users/'+id,data);
}
Create function and array in edit component to interact with services and api
src\app\components\employee-edit\employee-edit.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { DataService } from 'src/app/service/data.service';
import { Employee } from 'src/app/employee';
@Component({
selector: 'app-employee-edit',
templateUrl: './employee-edit.component.html',
styleUrls: ['./employee-edit.component.css']
})
export class EmployeeEditComponent implements OnInit {
id:any;
data:any;
employee = new Employee();
constructor(private route:ActivatedRoute, private dataService: DataService){}
ngOnInit(){
console.log(this.route.snapshot.params.id);
this.id = this.route.snapshot.params.id;
this.getData();
}
getData(){
this.dataService.getEmployeeByID(this.id).subscribe(res => {
this.data = res;
this.employee = this.data;
});
}
}
Import the activated route inside employee-edit.component.ts
src\app\components\employee-edit\employee-edit.component.ts
Create html form for update records
src\app\components\employee-edit\employee-edit.component.html
<form >
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<button class="btn btn-primary btn-sm mt-4">Update</button>
</form>
Delete Records :
Create a delete button in employee component html page
src\app\components\employee\employee.component.html
<button class="btn btn-danger btn-sm mz-2" (click)="deleteData(emp.id)">Delete</button>
Create Delete function in employee component ts file
src\app\components\employee\employee.component.ts
deleteData(id:any){
this.dataService.deleteData(id).subscribe(res => {
this.getEmployeesdata();
})
}
Call delete API inside data service ts file
src\app\service\data.service.ts
deleteData(id:any) {
return this.httpclient.delete('http://localhost:8000/api/users/'+id);
}
Complete Code :
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmployeeComponent } from './components/employee/employee.component';
import { NavbarComponent } from './components/navbar/navbar.component';
import { HttpClientModule } from '@angular/common/http';
import { FormsModule } from '@angular/forms';
import { EmployeeEditComponent } from './components/employee-edit/employee-edit.component';
const appRoutes : Routes = [
{path: '', component:EmployeeComponent},
{path: 'edit/:id', component:EmployeeEditComponent}
];
@NgModule({
declarations: [
AppComponent,
EmployeeComponent,
NavbarComponent,
EmployeeEditComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FormsModule,
RouterModule.forRoot(appRoutes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\app\components\employee\employee.component.ts
import { Component, OnInit } from '@angular/core';
import { Employee } from 'src/app/employee';
import { DataService } from 'src/app/service/data.service';
@Component({
selector: 'app-employee',
templateUrl: './employee.component.html',
styleUrls: ['./employee.component.css']
})
export class EmployeeComponent implements OnInit {
// export class EmployeeComponent {
employees:any;
employee = new Employee();
constructor(private dataService:DataService) { }
ngOnInit(){
this.getEmployeesdata();
}
getEmployeesdata() {
this.dataService.getData().subscribe(res =>{
this.employees = res;
});
}
deleteData(id:any){
this.dataService.deleteData(id).subscribe(res => {
this.getEmployeesdata();
})
}
insertData(){
this.dataService.insertData(this.employee).subscribe(res =>{
this.getEmployeesdata();
});
}
}
src\app\components\employee\employee.component.html
<form (ngSubmit)="insertData()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<div class="form-group">
<label for="name">Enter Password</label>
<input type="password" name="password" class="form-control" [(ngModel)]="employee.password">
</div>
<button class="btn btn-primary btn-sm mt-4">Submit</button>
</form>
<table class="table">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Email</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let emp of employees">
<td>{{ emp.id }}</td>
<td>{{ emp.name }}</td>
<td>{{ emp.email }}</td>
<td>
<button class="btn btn-warning btn-sm mx-2" routerLink="edit/{{ emp.id }}">Edit</button>
<button class="btn btn-danger btn-sm mz-2" (click)="deleteData(emp.id)">Delete</button>
</td>
</tr>
</tbody>
</table>
src\app\components\navbar\navbar.component.html
<nav class="navbar navbar-expand-lg bg-body-tertiary">
<div class="container-fluid">
<a class="navbar-brand" href="#">CRUD</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#">Home</a>
</li>
</ul>
</div>
</div>
</nav>
src\app\service\data.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private httpclient: HttpClient) { }
httpOptions = {
headers: new HttpHeaders({
'Content-Type': 'application/json',
}),
};
getData(){
return this.httpclient.get('http://localhost:8000/api/users/');
}
insertData(data:any){
return this.httpclient.post('http://localhost:8000/api/users/',data);
}
deleteData(id:any) {
return this.httpclient.delete('http://localhost:8000/api/users/'+id);
}
getEmployeeByID(id:any){
return this.httpclient.get('http://localhost:8000/api/users/'+id);
}
updateData(id:any,data:any){
return this.httpclient.put('http://localhost:8000/api/users/'+id, JSON.stringify(data),this.httpOptions);
}
}
src\app\employee.ts
export class Employee {
name:any;
email:any;
password:any;
}
src\app\components\employee-edit\employee-edit.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { DataService } from 'src/app/service/data.service';
import { Employee } from 'src/app/employee';
@Component({
selector: 'app-employee-edit',
templateUrl: './employee-edit.component.html',
styleUrls: ['./employee-edit.component.css']
})
export class EmployeeEditComponent implements OnInit {
id:any;
data:any;
employee = new Employee();
constructor(private route:ActivatedRoute, private dataService: DataService){}
ngOnInit(){
console.log(this.route.snapshot.params.id);
this.id = this.route.snapshot.params.id;
this.getData();
}
getData(){
this.dataService.getEmployeeByID(this.id).subscribe(res => {
this.data = res;
this.employee = this.data;
});
}
updateEmployee(){
this.dataService.updateData(this.id,this.employee).subscribe(res => {
})
}
}
src\app\components\employee-edit\employee-edit.component.html
<form (ngSubmit)="updateEmployee()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<button class="btn btn-primary btn-sm mt-4">Update</button>
</form>
Run Application :
ng serve --open
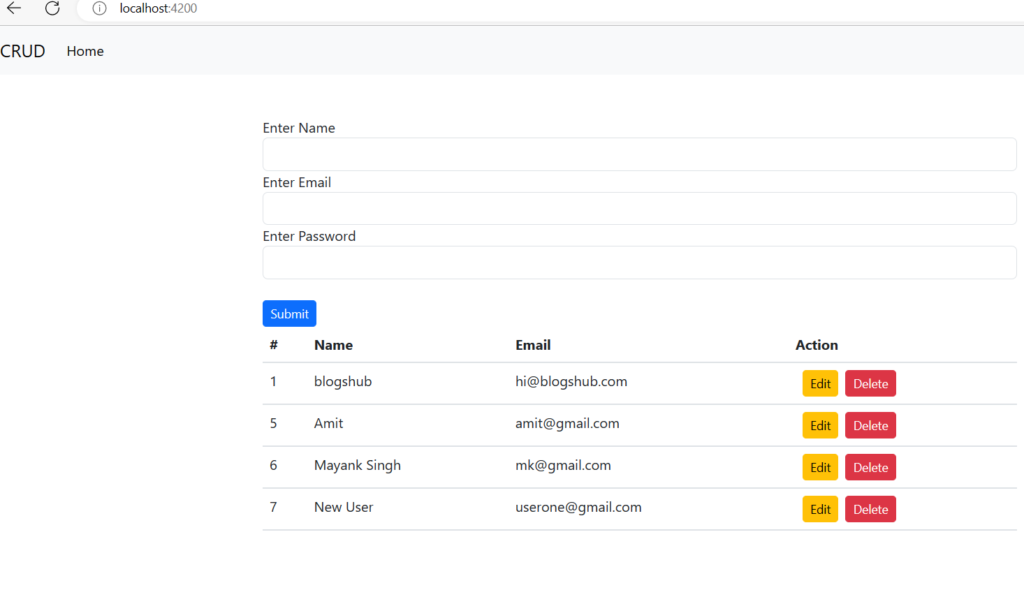
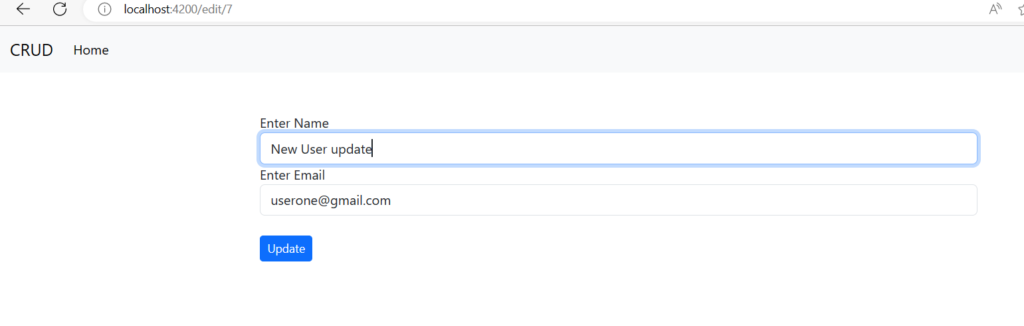
https://github.com/blogshub4/CRUDUSINGANGULAR
Keep Learning 🙂