How to Update or Edit data in database using Angular with API
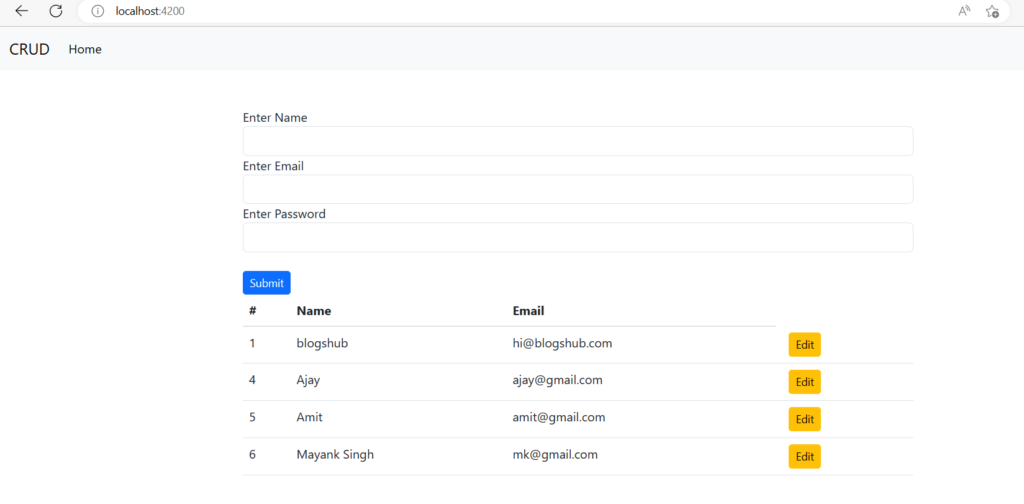
Hey Guys, in this post we will learn how to Update data in database using angular with API
for this post we will use laravel for backend API. First install Angular and Laravel
For installation insert and fetch record we have already another post you can follow those post using below links.
For installing Laravel and Creating CRUD API in Laravel you can follow below mention links
How to Install Laravel – BlogsHub
Create Laravel CRUD REST API – BlogsHub
How to Insert or Store data in database using Angular with API – BlogsHub
How to fetch data in Angular using API – BlogsHub
How to Create a new project in Angular – BlogsHub
Update Records Using Angular : First we have to create backend API , insert and fetch records using angular as mention above links, now we will updates the records
Create a edit component : For update records we have to create a component in angular using ng command as below.
ng g c components/employee-edit

Create edit button inside employee.component.html
<td> <button class="btn btn-warning btn-sm mx-2" routerLink="edit/{{ emp.id }}">Edit</button></td>
Create Route: Now we have to create a edit route inside app.module.ts file
src\app\app.module.ts
const appRoutes : Routes = [
{path: '', component:EmployeeComponent},
{path: 'edit/:id', component:EmployeeEditComponent}
];
Create functions in service file for fetching particular record by id and update records
src\app\service\data.service.ts
httpOptions = {
headers: new HttpHeaders({
'Content-Type': 'application/json',
}),
};
getEmployeeByID(id:any){
return this.httpclient.get('http://localhost:8000/api/users/'+id);
}
updateData(id:any,data:any){
return this.httpclient.put('http://localhost:8000/api/users/'+id, JSON.stringify(data),this.httpOptions);
}
Create function and array in edit component to interact with services and api
src\app\components\employee-edit\employee-edit.component.ts
getData(){
this.dataService.getEmployeeByID(this.id).subscribe(res => {
this.data = res;
this.employee = this.data;
});
}
updateEmployee(){
this.dataService.updateData(this.id,this.employee).subscribe(res => {
})
}
Create Form : Create html form for update records
src\app\components\employee-edit\employee-edit.component.html
<form (ngSubmit)="updateEmployee()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<button class="btn btn-primary btn-sm mt-4">Update</button>
</form>
Complete Code :
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmployeeComponent } from './components/employee/employee.component';
import { NavbarComponent } from './components/navbar/navbar.component';
import { HttpClientModule } from '@angular/common/http';
import { FormsModule } from '@angular/forms';
import { EmployeeEditComponent } from './components/employee-edit/employee-edit.component';
const appRoutes : Routes = [
{path: '', component:EmployeeComponent},
{path: 'edit/:id', component:EmployeeEditComponent}
];
@NgModule({
declarations: [
AppComponent,
EmployeeComponent,
NavbarComponent,
EmployeeEditComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FormsModule,
RouterModule.forRoot(appRoutes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\app\components\employee-edit\employee-edit.component.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { DataService } from 'src/app/service/data.service';
import { Employee } from 'src/app/employee';
@Component({
selector: 'app-employee-edit',
templateUrl: './employee-edit.component.html',
styleUrls: ['./employee-edit.component.css']
})
export class EmployeeEditComponent implements OnInit {
id:any;
data:any;
employee = new Employee();
constructor(private route:ActivatedRoute, private dataService: DataService){}
ngOnInit(){
console.log(this.route.snapshot.params.id);
this.id = this.route.snapshot.params.id;
this.getData();
}
getData(){
this.dataService.getEmployeeByID(this.id).subscribe(res => {
this.data = res;
this.employee = this.data;
});
}
updateEmployee(){
this.dataService.updateData(this.id,this.employee).subscribe(res => {
})
}
}
src\app\components\employee-edit\employee-edit.component.html
<form (ngSubmit)="updateEmployee()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<button class="btn btn-primary btn-sm mt-4">Update</button>
</form>
Run Application
ng serve --open
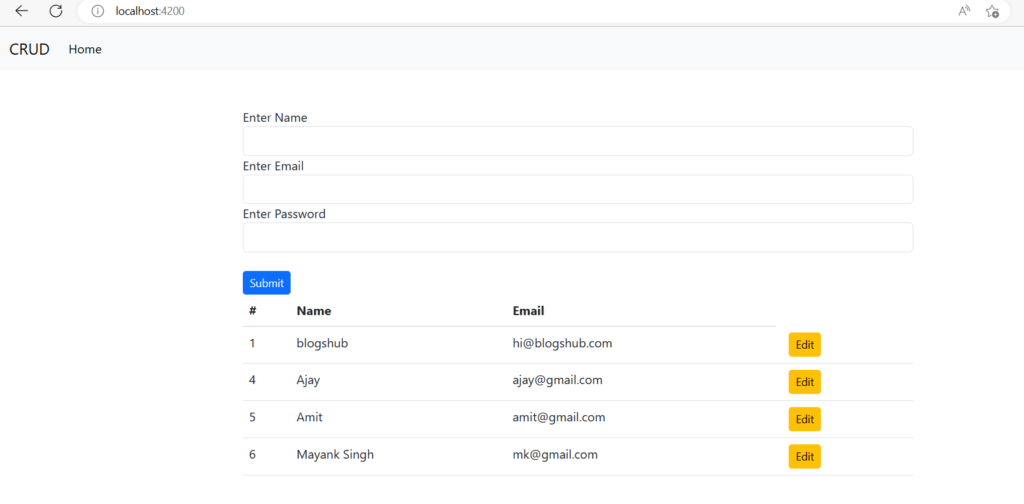
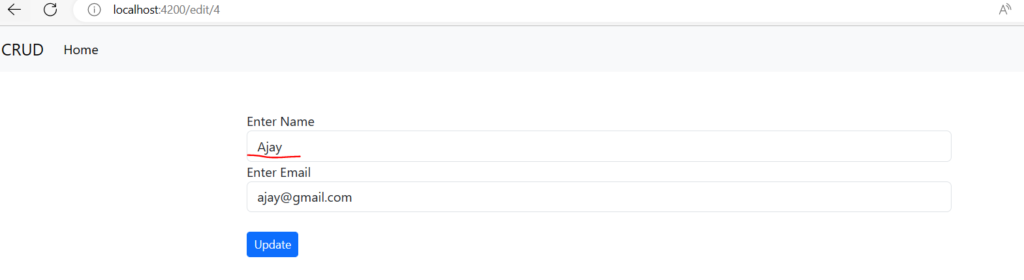
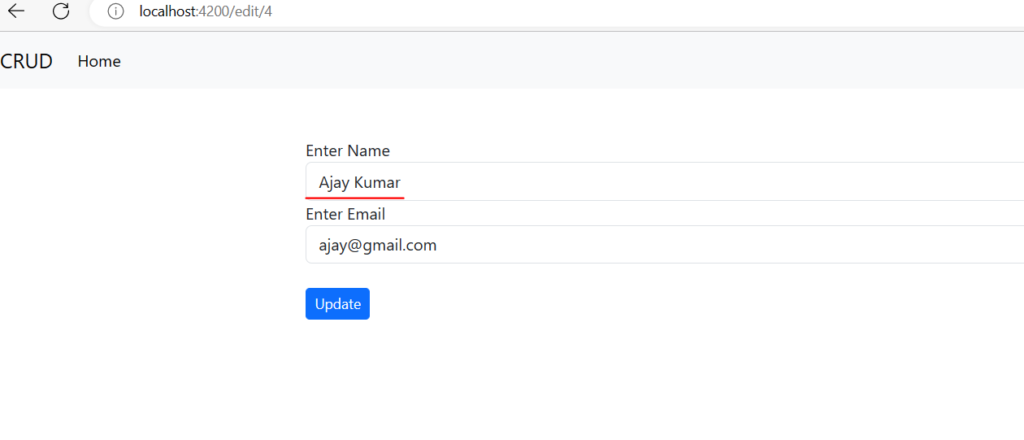
Happy Learing 🙂