How to fetch data in Angular using API
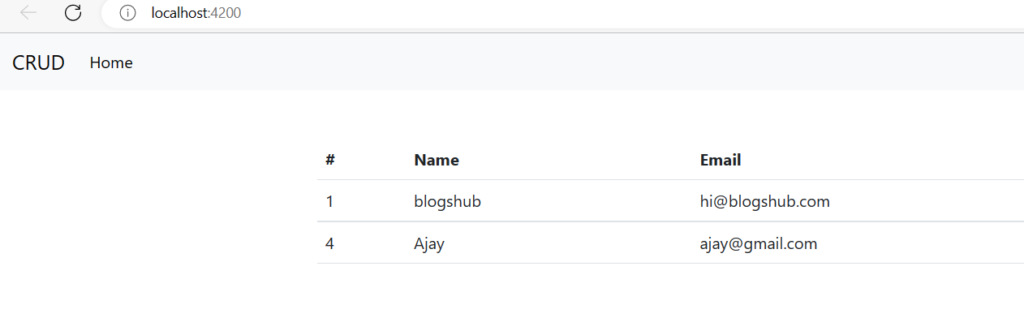
Hey Guys, in this post we will learn how to fetch data in angular using API
for this post we will use laravel for backend API. For fetching data we have to install Angular and Laravel
For installing Laravel and Creating CRUD API in Laravel you can also follow below mention links
Install Angular
We can install angular using npm command , open command prompt and run below command and press enter
npm install -g @angular/cli
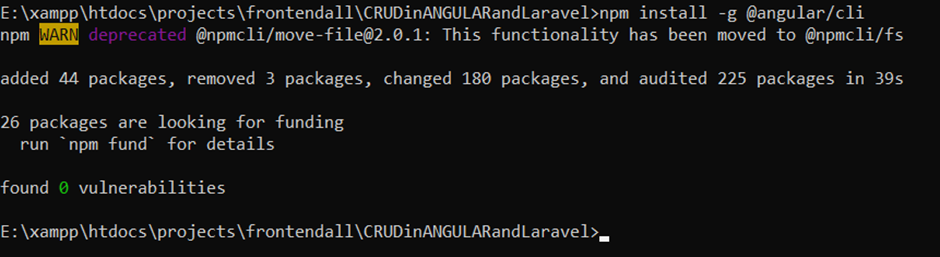
Now create new angular project or workspace, for that we have to run ng command as mention below
ng new frontcrudapp
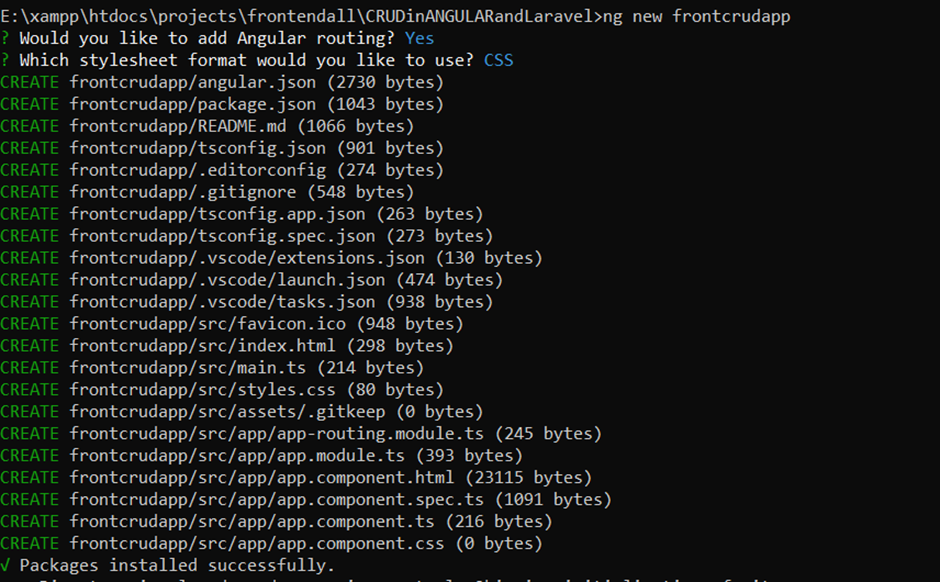
Now get in your application
cd frontcrudapp
Create Component : Lets create a component now , for creating a component we will use ng command.
ng g c components/employee
Above command will create a folder “components” and inside it will create components ,it will create five files .ts,.html and CSS files

Create Routes: routes are use for navigate the pages. For creating routes go inside app.module.ts and import routemodule, and then create route array and define routes path
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmployeeComponent } from './components/employee/employee.component';
import { NavbarComponent } from './components/navbar/navbar.component';
import { HttpClientModule } from '@angular/common/http';
const appRoutes : Routes = [
{path: '', component:EmployeeComponent}
];
@NgModule({
declarations: [
AppComponent,
EmployeeComponent,
NavbarComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
RouterModule.forRoot(appRoutes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
For accessing all API’s we have to create a service using ng command we can create services in angular. Using the services we can pass the data across components.
ng g s service/data

Open data service ts file for creating and accessing api
src\app\service\data.service.ts
import HttpClient
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private httpclient: HttpClient) { }
getData(){
return this.httpclient.get('http://localhost:8000/api/users/');
}
}
EmployeeComponent: open Employeecomponent ts file for defining array and function
(src\app\components\employee\employee.component.ts)
import { Component, OnInit } from '@angular/core';
import { DataService } from 'src/app/service/data.service';
@Component({
selector: 'app-employee',
templateUrl: './employee.component.html',
styleUrls: ['./employee.component.css']
})
export class EmployeeComponent implements OnInit {
// export class EmployeeComponent {
employees:any;
constructor(private dataService:DataService) { }
ngOnInit(){
this.getEmployeesdata();
}
getEmployeesdata() {
this.dataService.getData().subscribe(res =>{
this.employees = res;
});
}
}
Add bootstrap CSS in index.html
src\index.html
<link href=”https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css” rel=”stylesheet”>
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Frontcrudapp</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<app-root></app-root>
</body>
</html>
Navbar: Create a navbar component for creating a navbar in our application using ng command.
ng g c components/navbar

<nav class="navbar navbar-expand-lg bg-body-tertiary">
<div class="container-fluid">
<a class="navbar-brand" href="#">CRUD</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#">Home</a>
</li>
</ul>
</div>
</div>
</nav>
Fetch data in employeecomponent html file
src\app\components\employee\employee.component.html
<table class="table">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let emp of employees">
<td>{{ emp.id }}</td>
<td>{{ emp.name }}</td>
<td>{{ emp.email }}</td>
</tr>
</tbody>
</table>
Run your application
ng serve --open
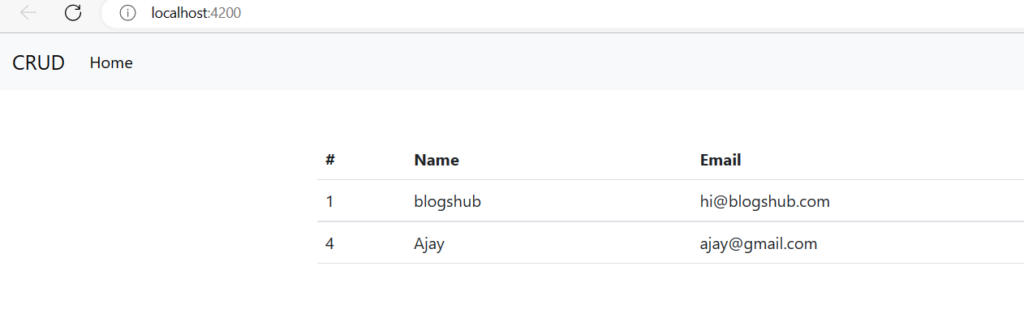
Keep Learning 🙂