How to Insert or Store data in database using Angular with API

Hey Guys, in this post we will learn how to insert data in database using angular with API
for this post we will use laravel for backend API. For inserting data we have to install Angular and Laravel
For installing Laravel and Creating CRUD API in Laravel you can follow below mention links
How to Install Laravel – BlogsHub
Create Laravel CRUD REST API – BlogsHub
How to fetch data in Angular using API – BlogsHub
Step 1: Install Angular
We can install angular using npm command , open command prompt and run below mention command and press enter.
npm install -g @angular/cli
After installing angular we have to create a angular application, we will create a new angular application with the ng command as mention below
ng new frontcrudapp
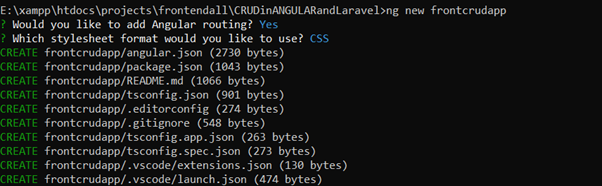
Now get in your application
cd frontcrudapp
Create Component : Now create a new component using below ng command.
ng g c components/employee

Routes: Create routes inside app.module.ts and import routemodule, and then create route array and define routes path
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmployeeComponent } from './components/employee/employee.component';
const appRoutes : Routes = [
{path: '', component:EmployeeComponent}
];
@NgModule({
declarations: [
AppComponent,
EmployeeComponent
],
imports: [
BrowserModule,
AppRoutingModule,
RouterModule.forRoot(appRoutes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Service: For communicating with API and components we have to create services.Run below command for creating service.
ng g s service/data

Create insertData function inside data.service.ts
src\app\service\data.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private httpclient: HttpClient) { }
insertData(data:any){
return this.httpclient.post('http://localhost:8000/api/users/',data);
}
}
Open employeecomponent and create insertData function for passing data to service.
(src\app\components\employee\employee.component.ts)
import { Component, OnInit } from '@angular/core';
import { Employee } from 'src/app/employee';
import { DataService } from 'src/app/service/data.service';
@Component({
selector: 'app-employee',
templateUrl: './employee.component.html',
styleUrls: ['./employee.component.css']
})
export class EmployeeComponent implements OnInit {
employee = new Employee();
constructor(private dataService:DataService) { }
ngOnInit(){
}
insertData(){
this.dataService.insertData(this.employee).subscribe(res =>{
});
}
}
Add bootstrap CSS in index.html
src\index.html
<link href=”https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css” rel=”stylesheet”>
Create Form:
For submit the data using angular we have to create a html form
So inside employee.component.html we will create a form
<form (ngSubmit)="insertData()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<div class="form-group">
<label for="name">Enter Password</label>
<input type="password" name="password" class="form-control" [(ngModel)]="employee.password">
</div>
<button class="btn btn-primary btn-sm mt-4">Submit</button>
</form>
Now we have to import the FormModule inside app.module.ts
src\app\app.module.ts
import { FormsModule } from '@angular/forms';
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FormsModule,
RouterModule.forRoot(appRoutes)
],
Then we have to call insertData function inside employee.component.ts, which we are using with HTML form.
src\app\components\employee\employee.component.ts
insertData(){
this.dataService.insertData(this.employee).subscribe(res =>{
this.getEmployeesdata();
});
Now create a model using below ng command in this model (employee.ts) we will define all input variable which we are getting to form like : name, email, password, it is helpful for two way data binding, using [(ngModel)] we can pass these model data type in html form.
ng g class Employee

export class Employee {
name:any;
email:any;
password:any;
}
We have to call/import Employee model inside employee.component.ts for creating object
import { Employee } from 'src/app/employee';
employee = new Employee();
Create insertData function in data.service.ts file for communicating with backend API
src\app\service\data.service.ts
insertData(data){
return this.httpclient.post('http://localhost:8000/api/users/',data);
}
Complete Code :
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { EmployeeComponent } from './components/employee/employee.component';
import { NavbarComponent } from './components/navbar/navbar.component';
import { HttpClientModule } from '@angular/common/http';
import { FormsModule } from '@angular/forms';
const appRoutes : Routes = [
{path: '', component:EmployeeComponent}
];
@NgModule({
declarations: [
AppComponent,
EmployeeComponent,
NavbarComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FormsModule,
RouterModule.forRoot(appRoutes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\app\components\employee\employee.component.ts
import { Component, OnInit } from '@angular/core';
import { Employee } from 'src/app/employee';
import { DataService } from 'src/app/service/data.service';
@Component({
selector: 'app-employee',
templateUrl: './employee.component.html',
styleUrls: ['./employee.component.css']
})
export class EmployeeComponent implements OnInit {
// export class EmployeeComponent {
employees:any;
employee = new Employee();
constructor(private dataService:DataService) { }
ngOnInit(){
this.getEmployeesdata();
}
getEmployeesdata() {
this.dataService.getData().subscribe(res =>{
this.employees = res;
});
}
insertData(){
this.dataService.insertData(this.employee).subscribe(res =>{
this.getEmployeesdata();
});
}
}
src\app\employee.ts
export class Employee {
name:any;
email:any;
password:any;
}
src\app\service\data.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private httpclient: HttpClient) { }
getData(){
return this.httpclient.get('http://localhost:8000/api/users/');
}
insertData(data:any){
return this.httpclient.post('http://localhost:8000/api/users/',data);
}
}
src\app\components\employee\employee.component.html
<form (ngSubmit)="insertData()">
<div class="form-group">
<label for="name">Enter Name</label>
<input type="text" name="name" class="form-control" [(ngModel)]="employee.name">
</div>
<div class="form-group">
<label for="name">Enter Email</label>
<input type="text" name="email" class="form-control" [(ngModel)]="employee.email">
</div>
<div class="form-group">
<label for="name">Enter Password</label>
<input type="password" name="password" class="form-control" [(ngModel)]="employee.password">
</div>
<button class="btn btn-primary btn-sm mt-4">Submit</button>
</form>
<table class="table">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let emp of employees">
<td>{{ emp.id }}</td>
<td>{{ emp.name }}</td>
<td>{{ emp.email }}</td>
</tr>
</tbody>
</table>
src\app\app.component.html
<div class="container">
<div class="row">
<div class="col mt-5 mb-5">
<div class="col-md-8 mx-auto">
<router-outlet></router-outlet>
</div>
</div>
</div>
</div>
Run application
ng serve --open

Keep Learning 🙂