Form Validation in angular
Hey guys in this post we learn how apply validation on HTML form using angular.
- Create angular project.
For creating a new angular project please follow the below mention link:
How to Create a new project in Angular – BlogsHub
2. Install bootstrap
We will use npm command for installation bootstrap in angular.
npm install bootstrap
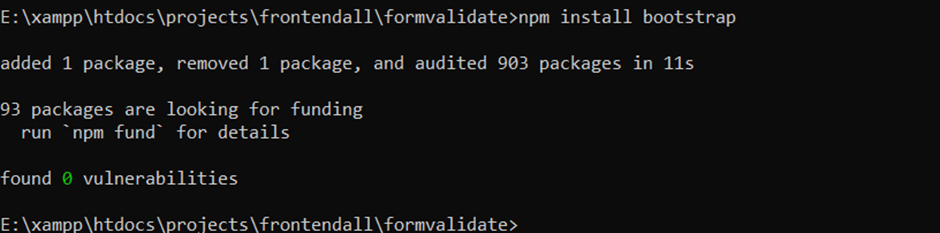
3. Add CSS
Now add css file path inside angular.json file in style array.
"styles": [
"src/styles.css",
"node_modules/bootstrap/dist/css/bootstrap.min.css"
],
4. Create Form
Now create a form in component file, eg: src\app\app.component.html
<div class="container">
<form>
<div class="mb-3">
<label for="exampleInputname1" class="form-label">Name</label>
<input type="text" class="form-control" id="exampleInputname1">
</div>
<div class="mb-3">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
Create form id and a function in form tag.
<form #myForm="ngForm" (ngSubmit)="formSubmit()">
5. Create Function
Now in src\app\app.component.ts create function which you are using with form.
formSubmit(){
console.log("Form working fine!")
}
6. Create Model
Create a model using ng command, model helps to store form data and send it to backend.
ng g class user –type=model

Define fields inside model as per your HTML form.
E:\xampp\htdocs\projects\frontendall\formvalidate\src\app\user.model.ts
export class User {
name:any;
email:any
}
Import user model inside component and create object
src\app\app.component.ts
import { User } from './user.model';
userobj = new User();
7. Define ngModel
Now define ngModel inside form fields.
<input type="text" class="form-control" id="exampleInputname1" #myName="ngModel" name="name" [(ngModel)]="userobj.name" required>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" #myEmail="ngModel" name="email" [(ngModel)]="userobj.email" required email>
8. Create validation statement
<span *ngIf="myName.invalid && myName.touched" class="text-warning">Invalid name</span>
<span *ngIf="myEmail.invalid && myEmail.touched" class="text-warning">Invalid email</span>
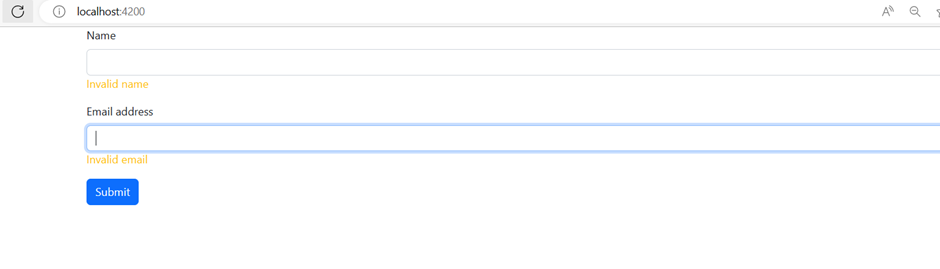
Complete Code:
src\app\app.component.html
<div class="container">
<form #myForm="ngForm" (ngSubmit)="formSubmit()">
<div class="mb-3">
<label for="exampleInputname1" class="form-label">Name</label>
<input type="text" class="form-control" id="exampleInputname1" #myName="ngModel" name="name" [(ngModel)]="userobj.name" pattern="[a-zA-Z]" required minlength="4" >
<span *ngIf="myName.invalid && myName.touched" class="text-warning">Invalid name</span>
</div>
<div class="mb-3">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" #myEmail="ngModel" name="email" [(ngModel)]="userobj.email" required email>
<span *ngIf="myEmail.invalid && myEmail.touched" class="text-warning">Invalid email</span>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
ReactiveFormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\app\app.component.ts
import { Component } from '@angular/core';
import { User } from './user.model';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'formvalidate';
userobj = new User();
formSubmit(){
console.log("Form working fine!")
}
}
src\app\user.model.ts
export class User {
name:any;
email:any
}
Keep Learning 🙂