How to Create Calendar and event in Angular
Hey guys in this post we will learn how to create a calendar and event in angular.
- Install Angular
- Create new project
For installing angular and creating new project follow below link.
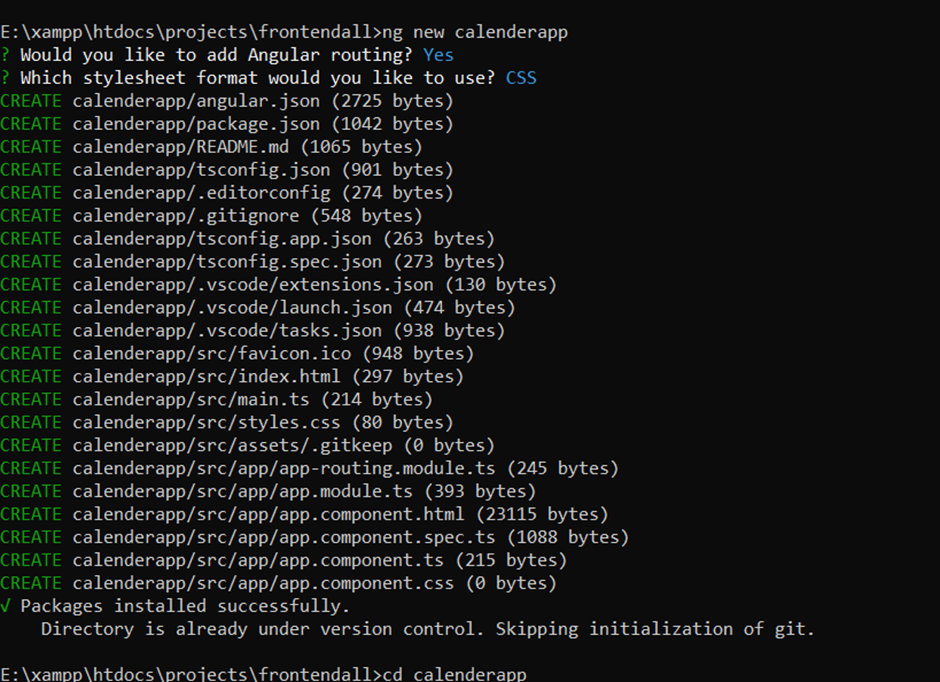
After creating project get in you project using cd command and run below commands for fullcalendar library
npm install --save @fullcalendar/core @fullcalendar/angular @fullcalendar/daygrid

Now open the appmodules.ts and import fullcalendar module.
import { FullCalendarModule } from '@fullcalendar/angular';
Inside import array add fullcalendar module in appmodules.ts file
imports:
FullCalendarModule
],
Inside appcomponent.ts file add below code
import { CalendarOptions } from '@fullcalendar/core'; // useful for typechecking
import dayGridPlugin from '@fullcalendar/daygrid';
In export class add below array object.
calendarOptions: CalendarOptions = {
initialView: 'dayGridMonth',
plugins: [dayGridPlugin]
};
Add fullcalendar tag in appcomponent.html
<full-calendar [options]="calendarOptions"></full-calendar>
In angular we can use bound input data versus outputs (angular has bount input and output concept)there is no distinction for fullcalendar connector between input and output. In this example we will pass into master options input as key value pair.
calendarOptions: CalendarOptions = {
initialView: 'dayGridMonth',
plugins: [dayGridPlugin],
events: [
{ title: 'event 1', date: '2023-03-12' },
{ title: 'event 2', date: '2023-03-14' }
]
};
Run your application.
ng serve --open
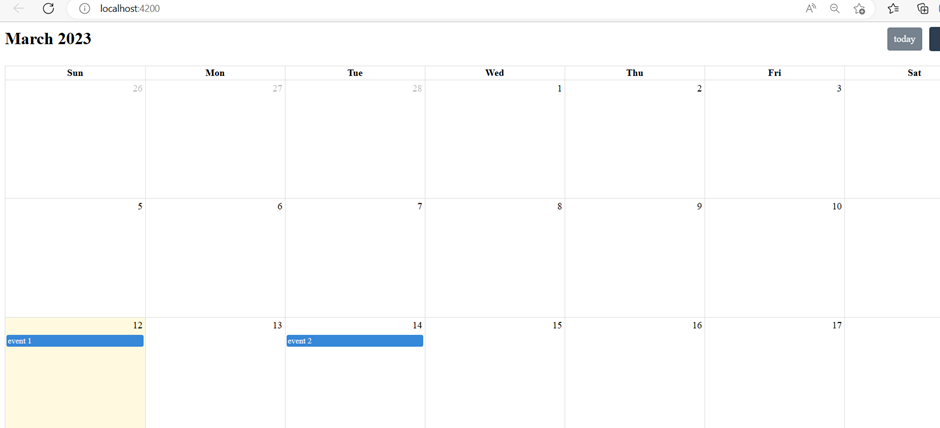
Complete Code
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FullCalendarModule } from '@fullcalendar/angular';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FullCalendarModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\app\app.component.ts
import { Component } from '@angular/core';
import { CalendarOptions } from '@fullcalendar/core'; // useful for typechecking
import dayGridPlugin from '@fullcalendar/daygrid';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'calenderapp';
calendarOptions: CalendarOptions = {
initialView: 'dayGridMonth',
plugins: [dayGridPlugin],
events: [
{ title: 'event 1', date: '2023-03-12' },
{ title: 'event 2', date: '2023-03-14' }
]
};
}
src\app\app.component.html
<full-calendar [options]="calendarOptions"></full-calendar>
<router-outlet></router-outlet>
Keep Learning 🙂