Pagination using angular with restful API
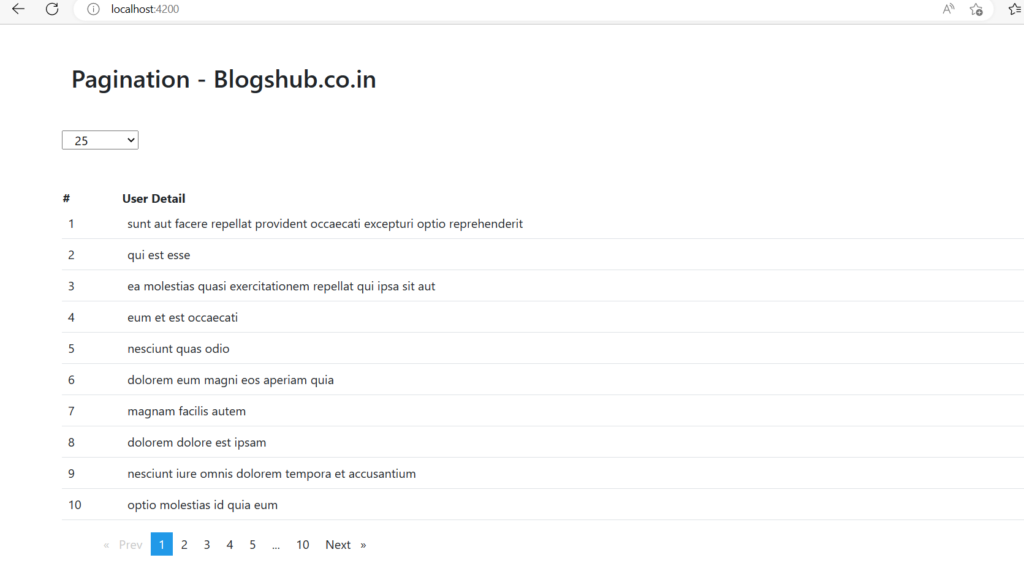
In this post we will learn how to create pagination using angular, for pagination we will create a new angular application using below steps.
Step 1: Install angular
ng new a_pagination
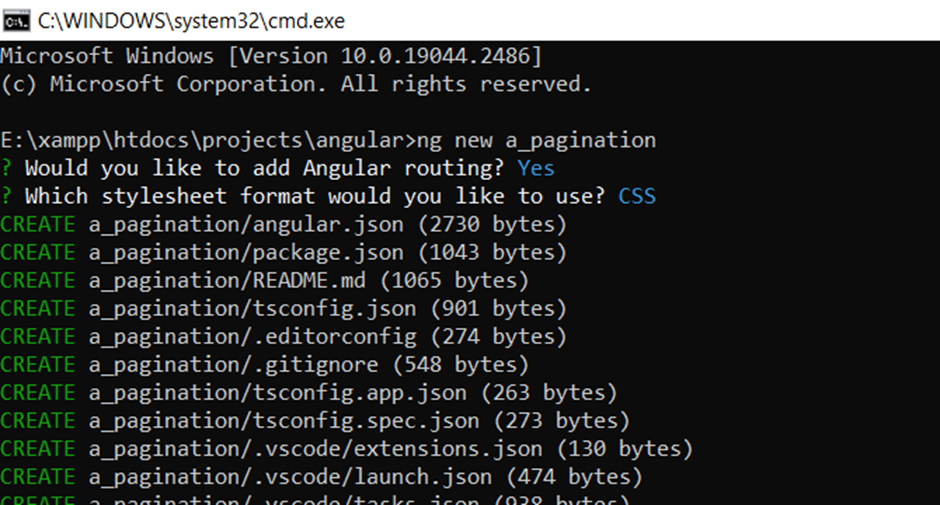
Get in application
cd a_pagination
Step 2 : Install ngx pagination library
Now we have to install ngx pagination library , that library we will call inside component, Run below command for install pagination library.
npm install ngx-pagination

Step 3: npm start
Now run npm start command
npm start
Step 4: import NgxPaginationModule and HttpClientModule
Now open app module ts and import NgxPaginationModule, HttpClientModule inside it
src\app\app.module.ts
import { NgxPaginationModule } from 'ngx-pagination';
import { HttpClientModule } from '@angular/common/http';
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
NgxPaginationModule
],
Step 5 : Add bootstrap css in index file.
Open index.html file and add bootstrap css.
src\index.html
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet">
Step 6 : Create Service
We have to create a service for communication with API, using below command we can create a service
ng generate service users
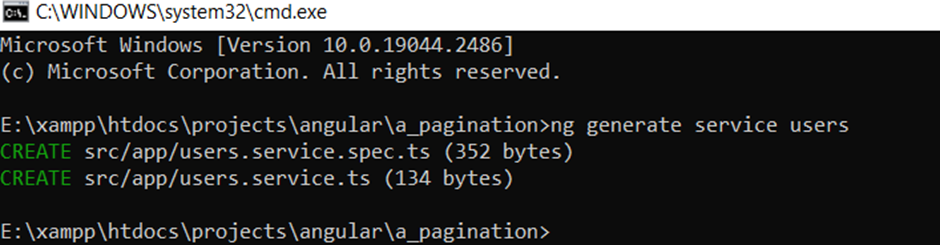
Open the service file and import Httpclient,Observable and create function for calling API
src\app\users.service.ts
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
getUsers() {
return this.http.get('https://jsonplaceholder.typicode.com/posts');
}
Then import the user service inside app component and create function.
src\app\app.component.ts
import { UsersService } from './users.service';
getUsers(){
this.userservice.getUsers().subscribe(res =>{
this.USERS = res;
console.log(this.USERS);
});
}
Now go inside component html page and display records
src\app\app.component.html
<div class="container">
<table class="table">
<thead>
<th>#</th>
<th>User Detail</th>
</thead>
<tbody>
<tr *ngFor="let user of USERS">
<td>{{ user.id }}</td>
<td>{{ user.title }}</td>
</tr>
</tbody>
</table>
</div>
Now create some more function for pagination in component ts file
src\app\app.component.ts
onTableDataChange(e:any){
this.page = e;
this.getUsers();
}
onSizeChange(e:any){
this.tableSize = e.target.value;
this.page = 1;
this.getUsers();
}
And now call pagination inside component html page
<tbody>
<tr *ngFor="let user of USERS | paginate:{itemsPerPage: tableSize, currentPage: page, totalItems: count}; let i index">
<td>{{ user.id }}</td>
<td>{{ user.title }}</td>
</tr>
</tbody>
</table>
<div>
<pagination-controls previousLabel="Prev" nextLabel="Next" (pageChange)="onTableDataChange($event)">
Complete Code
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { NgxPaginationModule } from 'ngx-pagination';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
NgxPaginationModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>APagination</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<app-root></app-root>
</body>
</html>
src\app\users.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class UsersService {
constructor(private http:HttpClient) { }
getUsers() {
return this.http.get('https://jsonplaceholder.typicode.com/posts');
}
}
src\app\app.component.ts
import { Component } from '@angular/core';
import { UsersService } from './users.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'a_pagination';
USERS:any;
page:number = 1;
count:number = 0;
tableSize:number = 10;
tableSizes:any = [10,15,20,25];
constructor (private userservice:UsersService){}
ngOnInit(){
this.getUsers();
}
getUsers(){
this.userservice.getUsers().subscribe(res =>{
this.USERS = res;
console.log(this.USERS);
});
}
onTableDataChange(e:any){
this.page = e;
this.getUsers();
}
onSizeChange(e:any){
this.tableSize = e.target.value;
this.page = 1;
this.getUsers();
}
}
src\app\app.component.html
<div class="container " >
<h2>Pagination - Blogshub.co.in</h2>
<div class="row" >
<select (change)="onSizeChange($event)" style="width:100px;" >
<option *ngFor ="let size of tableSizes">
{{ size }}
</option>
</select>
</div>
<div class="row" style="margin-top:50px;">
<table class="table">
<thead>
<th>#</th>
<th>User Detail</th>
</thead>
<tbody>
<tr *ngFor="let user of USERS | paginate:{itemsPerPage: tableSize, currentPage: page, totalItems: count}; let i index">
<td>{{ user.id }}</td>
<td>{{ user.title }}</td>
</tr>
</tbody>
</table>
<div>
<pagination-controls previousLabel="Prev" nextLabel="Next" (pageChange)="onTableDataChange($event)">
</pagination-controls>
</div>
</div>
</div>
Run Application :
ng serve --open
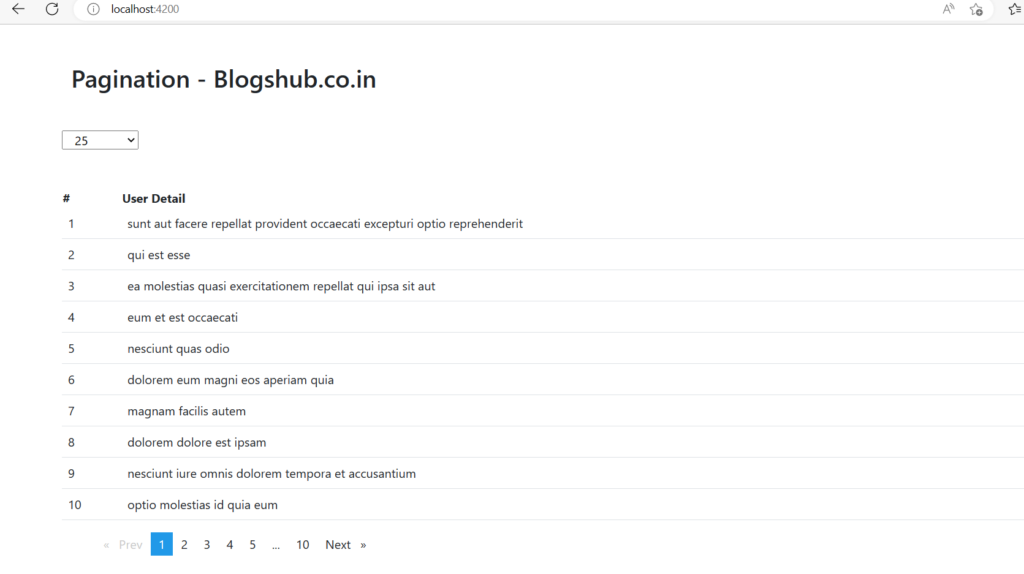
Keep Learning 🙂