How to import data in database with CSV and Seeder using laravel
In this post we will learn how to import CSV file data in databse using Laravel Seeder, and in the next post we will learn how to upload/import CSV data using form in databse.
Steps:
Step 1: Install Laravel and Create Project
Step 2: Config Database
Step 3: Create CSV File
Step 4: Create Model and Migrations
Step 5: Create Migrations
Step 6: Run Application
Create Laravel Application : We can use below composer command for creating laravel new application.
composer create-project --prefer-dist laravel/laravel seederwithCSV
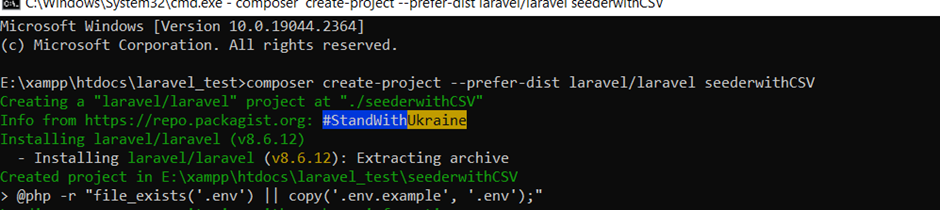
Get in laravel application
cd seederwithCSV

Config DataBase:
Create new database and config it using .env file
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=seederwithcsv
DB_USERNAME=root
DB_PASSWORD=
Create CSV File
database\csv\student.csv
Student_Name,Student_Class,Student_Rollno,Student_Phone
Ajay,I,1011,9885437665
Anuj,VI,1012,9145767341
Deepak,IV,1013,9848765658
Rahul,V,1014,9868737626
Tarun,III,1015,9828747617
Sohan,II,1016,9108367753
Deepika,X,1017,9278781659
Kavita,IX,1018,9348767451
Create Model : Create a model , using below cmd command we can create new model and migration file
php artisan make:model Student -m

<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Student extends Model
{
use HasFactory;
protected $fillable = [
'Student_Name',
'Student_Class',
'Student_Rollno',
'Student_Phone',
];
}
Now open migration file to database/migrations and create fields as per your requirements.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateStudentsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('students', function (Blueprint $table) {
$table->id();
$table->string('Student_Name');
$table->string('Student_Class');
$table->string('Student_Rollno');
$table->string('Student_Phone');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('students');
}
}
and then run below command for migrate your table in db
php artisan migrate
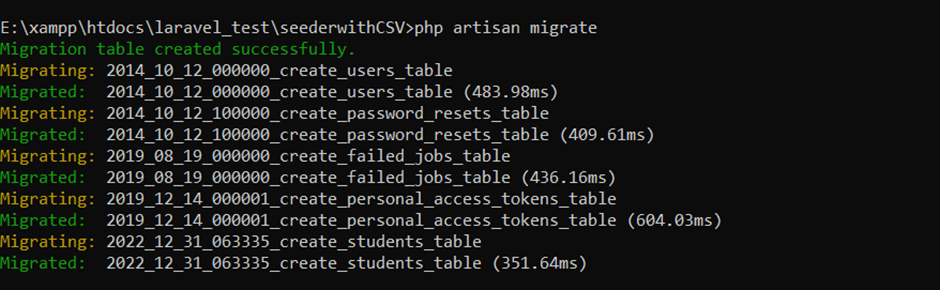
Now we have to create a seeder and call csv file inside seeder, run below command for creating seeder
php artisan make:seeder StudentSeeder

<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use App\Models\Student;
class StudentSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
Student::truncate();
$csvData = fopen(base_path('database/csv/student.csv'), 'r');
$transRow = true;
while (($data = fgetcsv($csvData, 555, ',')) !== false) {
if (!$transRow) {
Student::create([
'Student_Name' => $data['0'],
'Student_Class' => $data['1'],
'Student_Rollno' => $data['2'],
'Student_Phone' => $data['3'],
]);
}
$transRow = false;
}
fclose($csvData);
}
}
for insert all data run db seeder command
php artisan db:seed --class=StudentSeeder
Now check Database:
