How to Upload Image in Angular
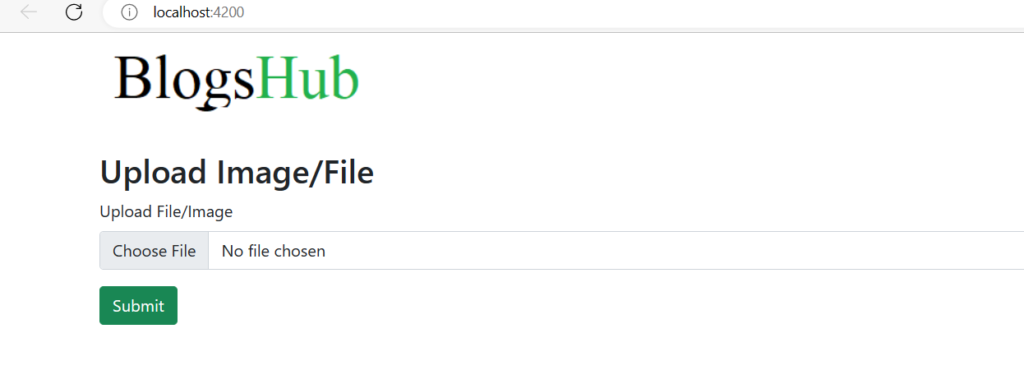
Hey guys in this post we will learn how to upload image or file in angular and store it in database , for this we have to create a backend API , for backend API please follow below post
Upload File in Laravel Using API with Postman – BlogsHub
Create a new application in angular , first you have to install angular then you can create new application using ng command
For installing angular follow below post
Create angular application :
ng new imageuploadfront
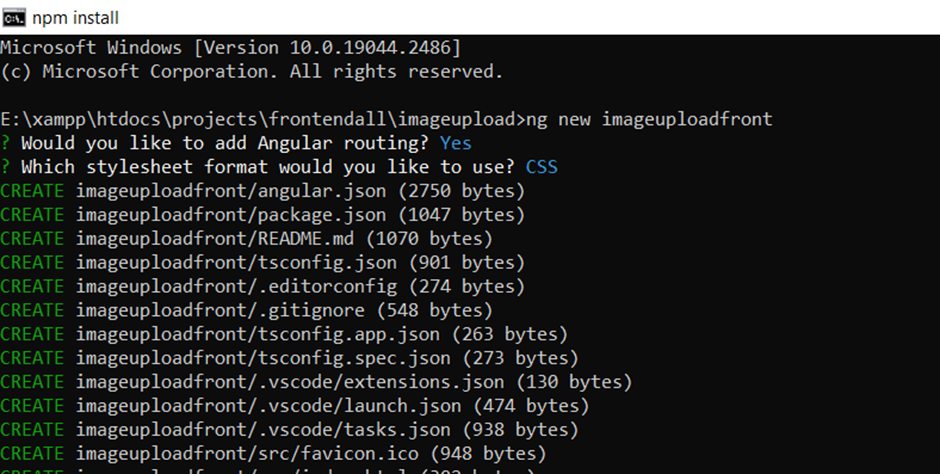
Get in application
cd imageuploadfront

For using bootsratp open index.html and add bootstrap CSS inside index file.
src\index.html
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
Create Form : For creating html form open app component html file and inside it create a form for upload file/image
src\app\app.component.html
<div class="container">
<h2>Upload Image</h2>
<form (ngSubmit)="onSubmit()" [formGroup]="form">
<div class="form-group">
<div class="mb-3">
<label for="UploadimgControlInput" class="form-label">Upload File/Image</label>
<input type="file" class="form-control" id="UploadimgControlInput" formControlName="image" name="image" (change)="uploadImage($event)" [ngClass]="{ 'is-invalid': submitted && f.image.errors }" placeholder="Upload Image">
<div *ngIf="submitted && f.image.errors" class="invalid-feedback">
<div *ngIf="f.image.errors.required">
Image is required
</div>
</div>
</div>
<div class="mb-3">
<input type="submit" class="btn btn-success">
</div>
</div>
</form>
</div>
Create a model: Create new model using below ng command and define fileds inside model.
ng g class Post --type=model

src\app\post.model.ts
export class Post {
image:any;
}
Create Component Functions: inside component ts file create functions for uploading image or file , and import model and related library in component file (formgroup , formbuilder and Post model).
src\app\app.component.ts
import { FormGroup, Validators, FormBuilder } from '@angular/forms';
import { Post } from './post.model';
import { ToastrService } from 'ngx-toastr';
Install Toastr: To show the upload message we will install the toastr, using the npm command we can install the toastr, open the command prompt run below npm command.
npm install ngx-toastr –save
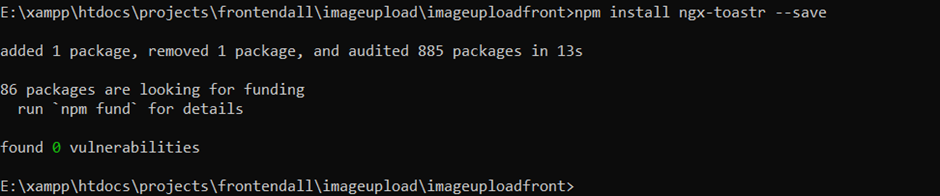
inside the angular.json file we have to add the toastr css file.
angular.json
"styles": [
"src/styles.css",
"node_modules/ngx-toastr/toastr.css"
],
Then import toastr service inside app.component.ts file
import { ToastrService } from 'ngx-toastr';
Now import formsmodule, reactiveformmodule, Browseranimationsmodule and toastrmodule inside app module ts file and then add all modules in import array inside app.module.ts.
src\app\app.module.ts
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { ToastrModule } from 'ngx-toastr';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
ReactiveFormsModule,
BrowserAnimationsModule
],
Create Service: For communicating between backend API and component functions we have to create a service, using the ng command we can create a service.
ng g s imageservice
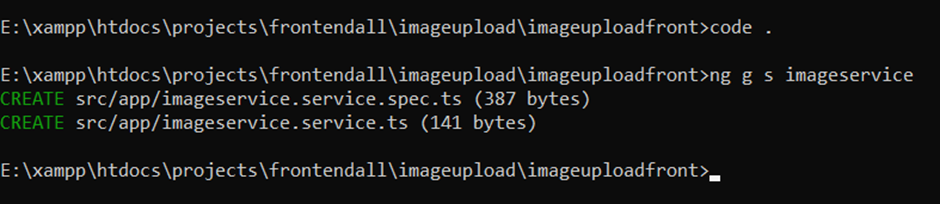
Open service file and import httpClient and httpHeader and create function for calling backend API.
src\app\imageservice.service.ts
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { environment } from 'src/environments/environment.development';
uploadImage(data:any){
const headers = new HttpHeaders();
return this.http.post(environment.apiUrl+'api/imgupload', data, {
headers:headers
});
API URL: Define API URL in environments file and then call that environment file inside service file (if environment file not exist you can create using ng command ex: ng generate environments)
src\environments\environment.ts
export const environment = {
apiUrl : "http://127.0.0.1:8000/"
};
Complete Code :
src\index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Imageuploadfront</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
</head>
<body>
<app-root></app-root>
</body>
</html>
src\app\app.component.html
<div class="container">
<h2>Upload Image</h2>
<form (ngSubmit)="onSubmit()" [formGroup]="form">
<div class="form-group">
<div class="mb-3">
<label for="UploadimgControlInput" class="form-label">Upload File/Image</label>
<input type="file" class="form-control" id="UploadimgControlInput" formControlName="image" name="image" (change)="uploadImage($event)" [ngClass]="{ 'is-invalid': submitted && f.image.errors }" placeholder="Upload Image">
<div *ngIf="submitted && f.image.errors" class="invalid-feedback">
<div *ngIf="f.image.errors.required">
Image is required
</div>
</div>
</div>
<div class="mb-3">
<input type="submit" class="btn btn-success">
</div>
</div>
</form>
</div>
src\app\app.component.ts
import { Component } from '@angular/core';
import { FormGroup, Validators, FormBuilder } from '@angular/forms';
import { Post } from './post.model';
import { ToastrService } from 'ngx-toastr';
import { ImageserviceService } from './imageservice.service';
// import { ToastrService } from 'ngx-toastr/public_api';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'imageuploadfront';
file:any;
submitted = false;
// form: FormGroup;
form: any;
post = new Post();
data:any;
constructor(private toastr:ToastrService, private formBuilder: FormBuilder, private imageservice:ImageserviceService) { }
ngOnInit(){
this.creatForm();
}
creatForm(){
this.form = this.formBuilder.group({
image: [null, Validators.required]
})
}
get f() {
return this.form.controls;
}
uploadImage(event:any){
this.file = event.target.files[0];
console.log(this.file);
}
onSubmit(){
this.submitted = true;
if(this.form.invalid){
return
}
const formData = new FormData();
formData.append("image", this.file, this.file.name);
this.imageservice.uploadImage(formData).subscribe(res => {
this.data = res;
if(this.data.status = true){
this.toastr.success(JSON.stringify(this.data.message),'',{
timeOut:2000,
progressBar:true
})
}
else {
this.toastr.error(JSON.stringify(this.data.message),'',{
timeOut:2000,
progressBar:true
})
}
this.submitted = false;
this.form.get('image').reset();
})
}
}
src\app\imageservice.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { environment } from 'src/environments/environment';
@Injectable({
providedIn: 'root'
})
export class ImageserviceService {
constructor(private http:HttpClient) { }
uploadImage(data:any){
const headers = new HttpHeaders();
return this.http.post(environment.apiUrl+'api/imgupload', data, {
headers:headers
});
}
}
src\environments\environment.ts
export const environment = {
apiUrl : "http://127.0.0.1:8000/"
};
src\app\app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { ToastrModule } from 'ngx-toastr';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
ReactiveFormsModule,
BrowserAnimationsModule,
HttpClientModule,
ToastrModule.forRoot()
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
src\app\post.model.ts
export class Post {
image:any;
}
Run Application:
ng serve –open
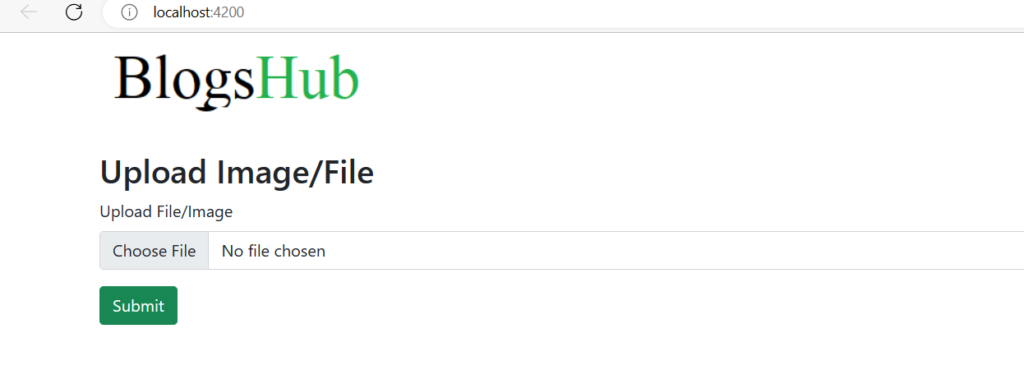
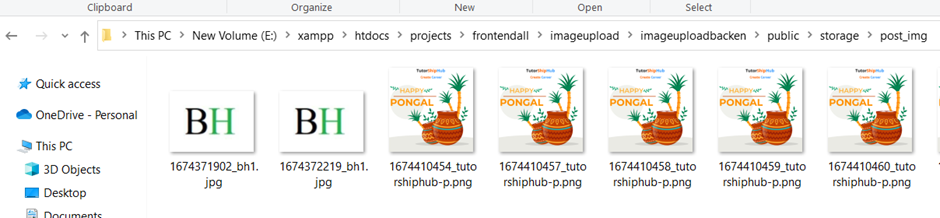
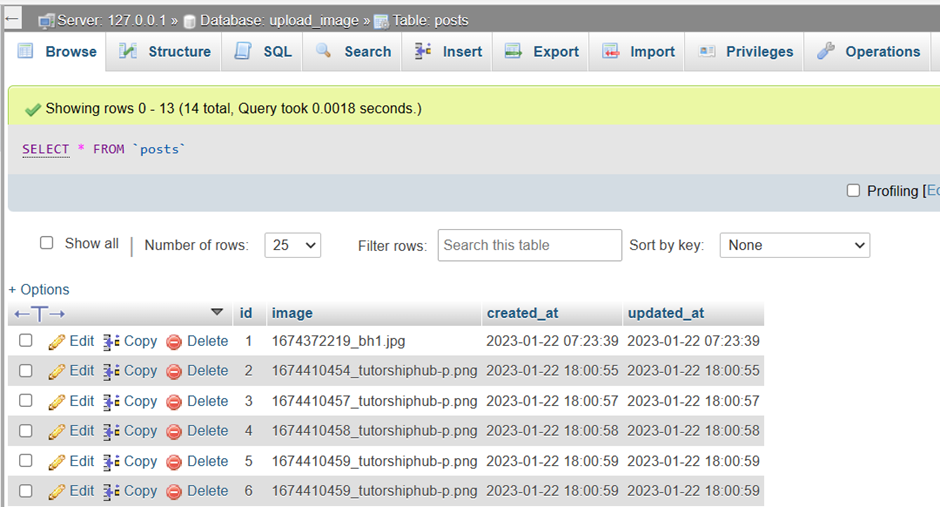
Keep Learning 🙂