Upload File in Laravel Using API with Postman
In this POST we will learn how to upload image or files via API in laravel.
We will use the following steps to upload image or files via API using postman in laravel.
Step 1: Install and Create Laravel Application
Step 2: Database Configuration using .env file
Step 3: Make Model and Migration file
Step 4: Make Controller
Step 5: Create Routes
Step 6: Create Folder
Step 7: Run Application
Step 8: Upload images/File using Postman
Step 9: View Complete Code
Install and Create Laravel Application
For creating new laravel application open command prompt and run below composer command
composer create-project --prefer-dist laravel/laravel imageuploadbacken
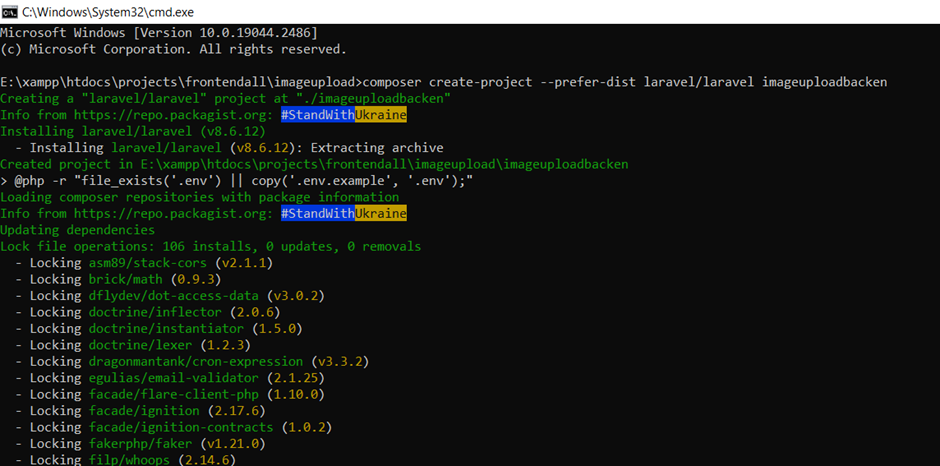
Get in application
Cd imageuploadbacken
Database Configuration using .env file
For databse configuration open .env file and set the database variables detail.

DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=upload_image
DB_USERNAME=root
DB_PASSWORD=
Make Model and Migration file
For model and migration run make:model artisan command as mention below it will create one model file and a migration file for table, then open your migratin file and add the image column
php artisan make:model Post -m

database\migrations\2023_01_22_062127_create_posts_table.php
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('image');
$table->timestamps();
});
}
app\Models\Post.php
Make the column fillable in post model
protected $fillable = ['image'];
now run migrate command to migrate all the tables with database.
php artisan migrate
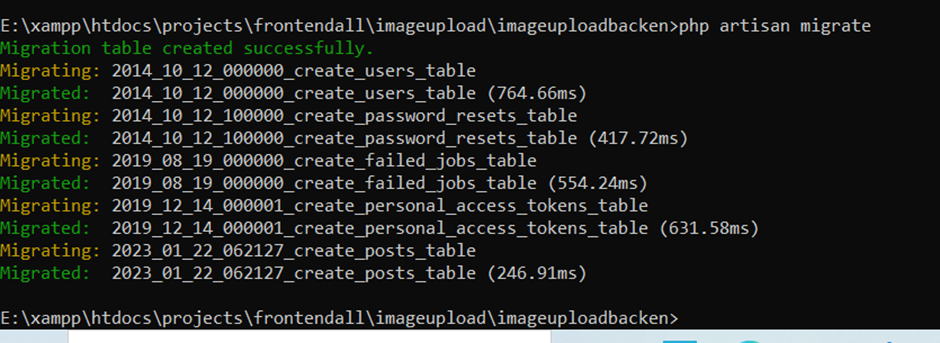
Make Controller
Create a new controller for upload file and create new function in it.
php artisan make:controller ImageController

<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
class ImageController extends Controller
{
public function imageUpload(Request $req) {
$postObj = new Post;
if($req->hasFile('image')) {
$filename = $req->file('image')->getClientOriginalName(); // get the file name
$getfilenamewitoutext = pathinfo($filename, PATHINFO_FILENAME); // get the file name without extension
$getfileExtension = $req->file('image')->getClientOriginalExtension(); // get the file extension
$createnewFileName = time().'_'.str_replace(' ','_', $getfilenamewitoutext).'.'.$getfileExtension; // create new random file name
$img_path = $req->file('image')->storeAs('public/post_img', $createnewFileName); // get the image path
$postObj->image = $createnewFileName; // pass file name with column
}
if($postObj->save()) { // save file in databse
return ['status' => true, 'message' => "Image uploded successfully"];
}
else {
return ['status' => false, 'message' => "Error : Image not uploded successfully"];
}
}
}
Create Routes
Create route inside api.php
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
use App\http\controllers\ImageController;
Route::post('imgupload', [ImageController::class, 'imageUpload']);
Create Folder
Create new folder for store images using below artisan command inside public directory and inside storage create “post_img” directory
php artisan storage:link

Run Application
php artisan serve
View Complete Code
database\migrations\2023_01_22_062127_create_posts_table.php
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('image');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
}
app\Models\Post.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
use HasFactory;
protected $fillable = ['image'];
}
app\Http\Controllers\ImageController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
class ImageController extends Controller
{
public function imageUpload(Request $req) {
$postObj = new Post;
if($req->hasFile('image')) {
$filename = $req->file('image')->getClientOriginalName(); // get the file name
$getfilenamewitoutext = pathinfo($filename, PATHINFO_FILENAME); // get the file name without extension
$getfileExtension = $req->file('image')->getClientOriginalExtension(); // get the file extension
$createnewFileName = time().'_'.str_replace(' ','_', $getfilenamewitoutext).'.'.$getfileExtension; // create new random file name
$img_path = $req->file('image')->storeAs('public/post_img', $createnewFileName); // get the image path
$postObj->image = $createnewFileName; // pass file name with column
}
if($postObj->save()) { // save file in databse
return ['status' => true, 'message' => "Image uploded successfully"];
}
else {
return ['status' => false, 'message' => "Error : Image not uploded successfully"];
}
}
}
routes\api.php
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
use App\http\controllers\ImageController;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
Route::post('imgupload', [ImageController::class, 'imageUpload']);
Now run api using postman , file field as mark in below screenshot then upload image in value column and click on send button



Keep Learning 🙂