How to Generate QR Code using Laravel
In this post we will learn how to generate QR Code using laravel.Laravel provides QR Code package which we will use, using it we can generate different kind of QR code. You have to install
Bacon/BaconQrCode package and then you have to register it with provider array inside config/app.php
Steps:
Step 1: Install Laravel
Step 2: Install QR Package
Step 3: Register QR Service
Step 4: Create one controller
Step 5: Create Route
Step 6: Create blade view file for QR Code
Step 7: Run Application
Install Laravel
Using composer below command we can install laravel , go in xampp, open CMD and run below command.
composer create-project --prefer-dist laravel/laravel qrcode

Get in laravel application
cd qrcode

Install QR Package
Run below command for installing QR Package with your application
composer require simplesoftwareio/simple-qrcode
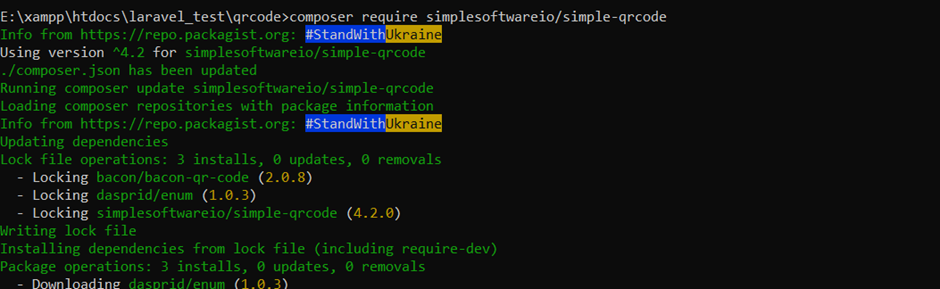
Inject QR service inside provider array inside config/app.php
'providers' => [
SimpleSoftwareIO\QrCode\QrCodeServiceProvider::class,
'aliases' => [
'QrCode' => SimpleSoftwareIO\QrCode\Facades\QrCode::class,
Create new controller using php artisan command
php artisan make:controller QrController
Create route in web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\QrController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get("qrcode",[QrController::class,'index']);
Create View file
Create one view file inside resources/views/viewqrcode.blade.php directory, and call it inside controller function.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class QrController extends Controller
{
public function index()
{
return view("viewqrcode");
}
}
Blade complete code
<!DOCTYPE html>
<html>
<head>
<title>Generate QR Using Laravel</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"/>
</head>
<body>
<h2> QR Code</h2>
<p>
{!! QrCode::size(200)->generate('RemoteStack') !!}
</p>
</body>
</html>
Now Run application using below command
php artisan serve
Access QR route
